The Unstable Book
Welcome to the Unstable Book! This book consists of a number of chapters, each one organized by a "feature flag." That is, when using an unstable feature of Rust, you must use a flag, like this:
#![feature(box_syntax)] fn main() { let five = box 5; }
The box_syntax
feature has a chapter describing how to use it.
Because this documentation relates to unstable features, we make no guarantees that what is contained here is accurate or up to date. It's developed on a best-effort basis. Each page will have a link to its tracking issue with the latest developments; you might want to check those as well.
Compiler flags
codegen-backend
The tracking issue for this feature is: #77933.
This feature allows you to specify a path to a dynamic library to use as rustc's code generation backend at runtime.
Set the -Zcodegen-backend=<path>
compiler flag to specify the location of the
backend. The library must be of crate type dylib
and must contain a function
named __rustc_codegen_backend
with a signature of fn() -> Box<dyn rustc_codegen_ssa::traits::CodegenBackend>
.
Example
See also the hotplug_codegen_backend
test
for a full example.
use rustc_codegen_ssa::traits::CodegenBackend;
struct MyBackend;
impl CodegenBackend for MyBackend {
// Implement codegen methods
}
#[no_mangle]
pub fn __rustc_codegen_backend() -> Box<dyn CodegenBackend> {
Box::new(MyBackend)
}
control-flow-guard
The tracking issue for this feature is: #68793.
The rustc flag -Z control-flow-guard
enables the Windows Control Flow Guard (CFG) platform security feature.
CFG is an exploit mitigation designed to enforce control-flow integrity for software running on supported Windows platforms (Windows 8.1 onwards). Specifically, CFG uses runtime checks to validate the target address of every indirect call/jump before allowing the call to complete.
During compilation, the compiler identifies all indirect calls/jumps and adds CFG checks. It also emits metadata containing the relative addresses of all address-taken functions. At runtime, if the binary is run on a CFG-aware operating system, the loader uses the CFG metadata to generate a bitmap of the address space and marks those addresses that contain valid targets. On each indirect call, the inserted check determines whether the target address is marked in this bitmap. If the target is not valid, the process is terminated.
In terms of interoperability:
- Code compiled with CFG enabled can be linked with libraries and object files that are not compiled with CFG. In this case, a CFG-aware linker can identify address-taken functions in the non-CFG libraries.
- Libraries compiled with CFG can linked into non-CFG programs. In this case, the CFG runtime checks in the libraries are not used (i.e. the mitigation is completely disabled).
CFG functionality is completely implemented in the LLVM backend and is supported for X86 (32-bit and 64-bit), ARM, and Aarch64 targets. The rustc flag adds the relevant LLVM module flags to enable the feature. This flag will be ignored for all non-Windows targets.
When to use Control Flow Guard
The primary motivation for enabling CFG in Rust is to enhance security when linking against non-Rust code, especially C/C++ code. To achieve full CFG protection, all indirect calls (including any from Rust code) must have the appropriate CFG checks, as added by this flag. CFG can also improve security for Rust code that uses the unsafe
keyword.
Another motivation behind CFG is to harden programs against return-oriented programming (ROP) attacks. CFG disallows an attacker from taking advantage of the program's own instructions while redirecting control flow in unexpected ways.
Overhead of Control Flow Guard
The CFG checks and metadata can potentially increase binary size and runtime overhead. The magnitude of any increase depends on the number and frequency of indirect calls. For example, enabling CFG for the Rust standard library increases binary size by approximately 0.14%. Enabling CFG in the SPEC CPU 2017 Integer Speed benchmark suite (compiled with Clang/LLVM) incurs approximate runtime overheads of between 0% and 8%, with a geometric mean of 2.9%.
Testing Control Flow Guard
The rustc flag -Z control-flow-guard=nochecks
instructs LLVM to emit the list of valid call targets without inserting runtime checks. This flag should only be used for testing purposes as it does not provide security enforcement.
Control Flow Guard in libraries
It is strongly recommended to also enable CFG checks for all linked libraries, including the standard library.
To enable CFG in the standard library, use the cargo -Z build-std
functionality to recompile the standard library with the same configuration options as the main program.
For example:
rustup toolchain install --force nightly
rustup component add rust-src
SET RUSTFLAGS=-Z control-flow-guard
cargo +nightly build -Z build-std --target x86_64-pc-windows-msvc
rustup toolchain install --force nightly
rustup component add rust-src
$Env:RUSTFLAGS = "-Z control-flow-guard"
cargo +nightly build -Z build-std --target x86_64-pc-windows-msvc
Alternatively, if you are building the standard library from source, you can set control-flow-guard = true
in the config.toml file.
`debug-info-for-profiling
Introduction
Automatic Feedback Directed Optimization (AFDO) is a method for using sampling based profiles to guide optimizations. This is contrasted with other methods of FDO or profile-guided optimization (PGO) which use instrumented profiling.
Unlike PGO (controlled by the rustc
flags -Cprofile-generate
and
-Cprofile-use
), a binary being profiled does not perform significantly worse,
and thus it's possible to profile binaries used in real workflows and not
necessary to construct artificial workflows.
Use
In order to use AFDO, the target platform must be Linux running on an x86_64
architecture with the performance profiler perf
available. In addition, the
external tool create_llvm_prof
from this repository must be used.
Given a Rust file main.rs
, we can produce an optimized binary as follows:
rustc -O -Zdebug-info-for-profiling main.rs -o main
perf record -b ./main
create_llvm_prof --binary=main --out=code.prof
rustc -O -Zprofile-sample-use=code.prof main.rs -o main2
The perf
command produces a profile perf.data
, which is then used by the
create_llvm_prof
command to create code.prof
. This final profile is then
used by rustc
to guide optimizations in producing the binary main2
.
emit-stack-sizes
The tracking issue for this feature is: #54192
The rustc flag -Z emit-stack-sizes
makes LLVM emit stack size metadata.
NOTE: This LLVM feature only supports the ELF object format as of LLVM 8.0. Using this flag with targets that use other object formats (e.g. macOS and Windows) will result in it being ignored.
Consider this crate:
#![crate_type = "lib"]
use std::ptr;
pub fn foo() {
// this function doesn't use the stack
}
pub fn bar() {
let xs = [0u32; 2];
// force LLVM to allocate `xs` on the stack
unsafe { ptr::read_volatile(&xs.as_ptr()); }
}
Using the -Z emit-stack-sizes
flag produces extra linker sections in the
output object file.
$ rustc -C opt-level=3 --emit=obj foo.rs
$ size -A foo.o
foo.o :
section size addr
.text 0 0
.text._ZN3foo3foo17he211d7b4a3a0c16eE 1 0
.text._ZN3foo3bar17h1acb594305f70c2eE 22 0
.note.GNU-stack 0 0
.eh_frame 72 0
Total 95
$ rustc -C opt-level=3 --emit=obj -Z emit-stack-sizes foo.rs
$ size -A foo.o
foo.o :
section size addr
.text 0 0
.text._ZN3foo3foo17he211d7b4a3a0c16eE 1 0
.stack_sizes 9 0
.text._ZN3foo3bar17h1acb594305f70c2eE 22 0
.stack_sizes 9 0
.note.GNU-stack 0 0
.eh_frame 72 0
Total 113
As of LLVM 7.0 the data will be written into a section named .stack_sizes
and
the format is "an array of pairs of function symbol values (pointer size) and
stack sizes (unsigned LEB128)".
$ objdump -d foo.o
foo.o: file format elf64-x86-64
Disassembly of section .text._ZN3foo3foo17he211d7b4a3a0c16eE:
0000000000000000 <_ZN3foo3foo17he211d7b4a3a0c16eE>:
0: c3 retq
Disassembly of section .text._ZN3foo3bar17h1acb594305f70c2eE:
0000000000000000 <_ZN3foo3bar17h1acb594305f70c2eE>:
0: 48 83 ec 10 sub $0x10,%rsp
4: 48 8d 44 24 08 lea 0x8(%rsp),%rax
9: 48 89 04 24 mov %rax,(%rsp)
d: 48 8b 04 24 mov (%rsp),%rax
11: 48 83 c4 10 add $0x10,%rsp
15: c3 retq
$ objdump -s -j .stack_sizes foo.o
foo.o: file format elf64-x86-64
Contents of section .stack_sizes:
0000 00000000 00000000 00 .........
Contents of section .stack_sizes:
0000 00000000 00000000 10 .........
It's important to note that linkers will discard this linker section by default. To preserve the section you can use a linker script like the one shown below.
/* file: keep-stack-sizes.x */
SECTIONS
{
/* `INFO` makes the section not allocatable so it won't be loaded into memory */
.stack_sizes (INFO) :
{
KEEP(*(.stack_sizes));
}
}
The linker script must be passed to the linker using a rustc flag like -C link-arg
.
// file: src/main.rs
use std::ptr;
#[inline(never)]
fn main() {
let xs = [0u32; 2];
// force LLVM to allocate `xs` on the stack
unsafe { ptr::read_volatile(&xs.as_ptr()); }
}
$ RUSTFLAGS="-Z emit-stack-sizes" cargo build --release
$ size -A target/release/hello | grep stack_sizes || echo section was not found
section was not found
$ RUSTFLAGS="-Z emit-stack-sizes" cargo rustc --release -- \
-C link-arg=-Wl,-Tkeep-stack-sizes.x \
-C link-arg=-N
$ size -A target/release/hello | grep stack_sizes
.stack_sizes 90 176272
$ # non-allocatable section (flags don't contain the "A" (alloc) flag)
$ readelf -S target/release/hello
Section Headers:
[Nr] Name Type Address Offset
Size EntSize Flags Link Info Align
(..)
[1031] .stack_sizes PROGBITS 000000000002b090 0002b0f0
000000000000005a 0000000000000000 L 5 0 1
$ objdump -s -j .stack_sizes target/release/hello
target/release/hello: file format elf64-x86-64
Contents of section .stack_sizes:
2b090 c0040000 00000000 08f00400 00000000 ................
2b0a0 00080005 00000000 00000810 05000000 ................
2b0b0 00000000 20050000 00000000 10400500 .... ........@..
2b0c0 00000000 00087005 00000000 00000080 ......p.........
2b0d0 05000000 00000000 90050000 00000000 ................
2b0e0 00a00500 00000000 0000 ..........
Author note: I'm not entirely sure why, in this case,
-N
is required in addition to-Tkeep-stack-sizes.x
. For example, it's not required when producing statically linked files for the ARM Cortex-M architecture.
extern-location
MCP for this feature: #303
The unused-extern-crates
lint reports when a crate was specified on the rustc
command-line with --extern name=path
but no symbols were referenced in it.
This is useful to know, but it's hard to map that back to a specific place a user
or tool could fix (ie, to remove the unused dependency).
The --extern-location
flag allows the build system to associate a location with
the --extern
option, which is then emitted as part of the diagnostics. This location
is abstract and just round-tripped through rustc; the compiler never attempts to
interpret it in any way.
There are two supported forms of location: a bare string, or a blob of json:
--extern-location foo=raw:Makefile:123
would associate the raw stringMakefile:123
--extern-location 'bar=json:{"target":"//my_project:library","dep":"//common:serde"}
would associate the json structure with--extern bar=<path>
, indicating which dependency of which rule introduced the unused extern crate.
This primarily intended to be used with tooling - for example a linter which can automatically remove unused dependencies - rather than being directly presented to users.
raw
locations are presented as part of the normal rendered diagnostics and included in
the json form. json
locations are only included in the json form of diagnostics,
as a tool_metadata
field. For raw
locations tool_metadata
is simply a json string,
whereas json
allows the rustc invoker to fully control its form and content.
instrument-coverage
The tracking issue for this feature is: #79121.
Introduction
The Rust compiler includes two code coverage implementations:
- A GCC-compatible, gcov-based coverage implementation, enabled with
-Z profile
, which derives coverage data based on DebugInfo. - A source-based code coverage implementation, enabled with
-Z instrument-coverage
, which uses LLVM's native, efficient coverage instrumentation to generate very precise coverage data.
This document describes how to enable and use the LLVM instrumentation-based coverage, via the -Z instrument-coverage
compiler flag.
How it works
When -Z instrument-coverage
is enabled, the Rust compiler enhances rust-based libraries and binaries by:
- Automatically injecting calls to an LLVM intrinsic (
llvm.instrprof.increment
), at functions and branches in compiled code, to increment counters when conditional sections of code are executed. - Embedding additional information in the data section of each library and binary (using the LLVM Code Coverage Mapping Format Version 4, supported only in LLVM 11 and up), to define the code regions (start and end positions in the source code) being counted.
When running a coverage-instrumented program, the counter values are written to a profraw
file at program termination. LLVM bundles tools that read the counter results, combine those results with the coverage map (embedded in the program binary), and generate coverage reports in multiple formats.
Note:
-Z instrument-coverage
also automatically enables-Z symbol-mangling-version=v0
(tracking issue #60705). Thev0
symbol mangler is strongly recommended, but be aware that this demangler is also experimental. Thev0
demangler can be overridden by explicitly adding-Z symbol-mangling-version=legacy
.
Enable coverage profiling in the Rust compiler
Rust's source-based code coverage requires the Rust "profiler runtime". Without it, compiling with -Z instrument-coverage
generates an error that the profiler runtime is missing.
The Rust nightly
distribution channel includes the profiler runtime, by default.
Important: If you are building the Rust compiler from the source distribution, the profiler runtime is not enabled in the default
config.toml.example
. Edit yourconfig.toml
file and ensure theprofiler
feature is set it totrue
(either under the[build]
section, or under the settings for an individual[target.<triple>]
):# Build the profiler runtime (required when compiling with options that depend # on this runtime, such as `-C profile-generate` or `-Z instrument-coverage`). profiler = true
Building the demangler
LLVM coverage reporting tools generate results that can include function names and other symbol references, and the raw coverage results report symbols using the compiler's "mangled" version of the symbol names, which can be difficult to interpret. To work around this issue, LLVM coverage tools also support a user-specified symbol name demangler.
One option for a Rust demangler is rustfilt
, which can be installed with:
cargo install rustfilt
Another option, if you are building from the Rust compiler source distribution, is to use the rust-demangler
tool included in the Rust source distribution, which can be built with:
$ ./x.py build rust-demangler
Compiling with coverage enabled
Set the -Z instrument-coverage
compiler flag in order to enable LLVM source-based code coverage profiling.
The default option generates coverage for all functions, including unused (never called) functions and generics. The compiler flag supports an optional value to tailor this behavior. (See -Z instrument-coverage=<options>
, below.)
With cargo
, you can instrument your program binary and dependencies at the same time.
For example (if your project's Cargo.toml builds a binary by default):
$ cd your-project
$ cargo clean
$ RUSTFLAGS="-Z instrument-coverage" cargo build
If cargo
is not configured to use your profiler
-enabled version of rustc
, set the path explicitly via the RUSTC
environment variable. Here is another example, using a stage1
build of rustc
to compile an example
binary (from the json5format
crate):
$ RUSTC=$HOME/rust/build/x86_64-unknown-linux-gnu/stage1/bin/rustc \
RUSTFLAGS="-Z instrument-coverage" \
cargo build --example formatjson5
Note: that some compiler options, combined with
-Z instrument-coverage
, can produce LLVM IR and/or linked binaries that are incompatible with LLVM coverage maps. For example, coverage requires references to actual functions in LLVM IR. If any covered function is optimized out, the coverage tools may not be able to process the coverage results. If you need to pass additional options, with coverage enabled, test them early, to confirm you will get the coverage results you expect.
Running the instrumented binary to generate raw coverage profiling data
In the previous example, cargo
generated the coverage-instrumented binary formatjson5
:
$ echo "{some: 'thing'}" | target/debug/examples/formatjson5 -
{
some: "thing",
}
After running this program, a new file, default.profraw
, should be in the current working directory. It's often preferable to set a specific file name or path. You can change the output file using the environment variable LLVM_PROFILE_FILE
:
$ echo "{some: 'thing'}" \
| LLVM_PROFILE_FILE="formatjson5.profraw" target/debug/examples/formatjson5 -
...
$ ls formatjson5.profraw
formatjson5.profraw
If LLVM_PROFILE_FILE
contains a path to a non-existent directory, the missing directory structure will be created. Additionally, the following special pattern strings are rewritten:
%p
- The process ID.%h
- The hostname of the machine running the program.%t
- The value of the TMPDIR environment variable.%Nm
- the instrumented binary’s signature: The runtime creates a pool of N raw profiles, used for on-line profile merging. The runtime takes care of selecting a raw profile from the pool, locking it, and updating it before the program exits.N
must be between1
and9
, and defaults to1
if omitted (with simply%m
).%c
- Does not add anything to the filename, but enables a mode (on some platforms, including Darwin) in which profile counter updates are continuously synced to a file. This means that if the instrumented program crashes, or is killed by a signal, perfect coverage information can still be recovered.
Installing LLVM coverage tools
LLVM's supplies two tools—llvm-profdata
and llvm-cov
—that process coverage data and generate reports. There are several ways to find and/or install these tools, but note that the coverage mapping data generated by the Rust compiler requires LLVM version 11 or higher. (llvm-cov --version
typically shows the tool's LLVM version number.):
- The LLVM tools may be installed (or installable) directly to your OS (such as via
apt-get
, for Linux). - If you are building the Rust compiler from source, you can optionally use the bundled LLVM tools, built from source. Those tool binaries can typically be found in your build platform directory at something like:
rust/build/x86_64-unknown-linux-gnu/llvm/bin/llvm-*
. - You can install compatible versions of these tools via
rustup
.
The rustup
option is guaranteed to install a compatible version of the LLVM tools, but they can be hard to find. We recommend cargo-binutils
, which installs Rust-specific wrappers around these and other LLVM tools, so you can invoke them via cargo
commands!
$ rustup component add llvm-tools-preview
$ cargo install cargo-binutils
$ cargo profdata -- --help # note the additional "--" preceding the tool-specific arguments
Creating coverage reports
Raw profiles have to be indexed before they can be used to generate coverage reports. This is done using llvm-profdata merge
(or cargo profdata -- merge
), which can combine multiple raw profiles and index them at the same time:
$ llvm-profdata merge -sparse formatjson5.profraw -o formatjson5.profdata
Finally, the .profdata
file is used, in combination with the coverage map (from the program binary) to generate coverage reports using llvm-cov report
(or cargo cov -- report
), for a coverage summaries; and llvm-cov show
(or cargo cov -- show
), to see detailed coverage of lines and regions (character ranges) overlaid on the original source code.
These commands have several display and filtering options. For example:
$ llvm-cov show -Xdemangler=rustfilt target/debug/examples/formatjson5 \
-instr-profile=formatjson5.profdata \
-show-line-counts-or-regions \
-show-instantiations \
-name=add_quoted_string
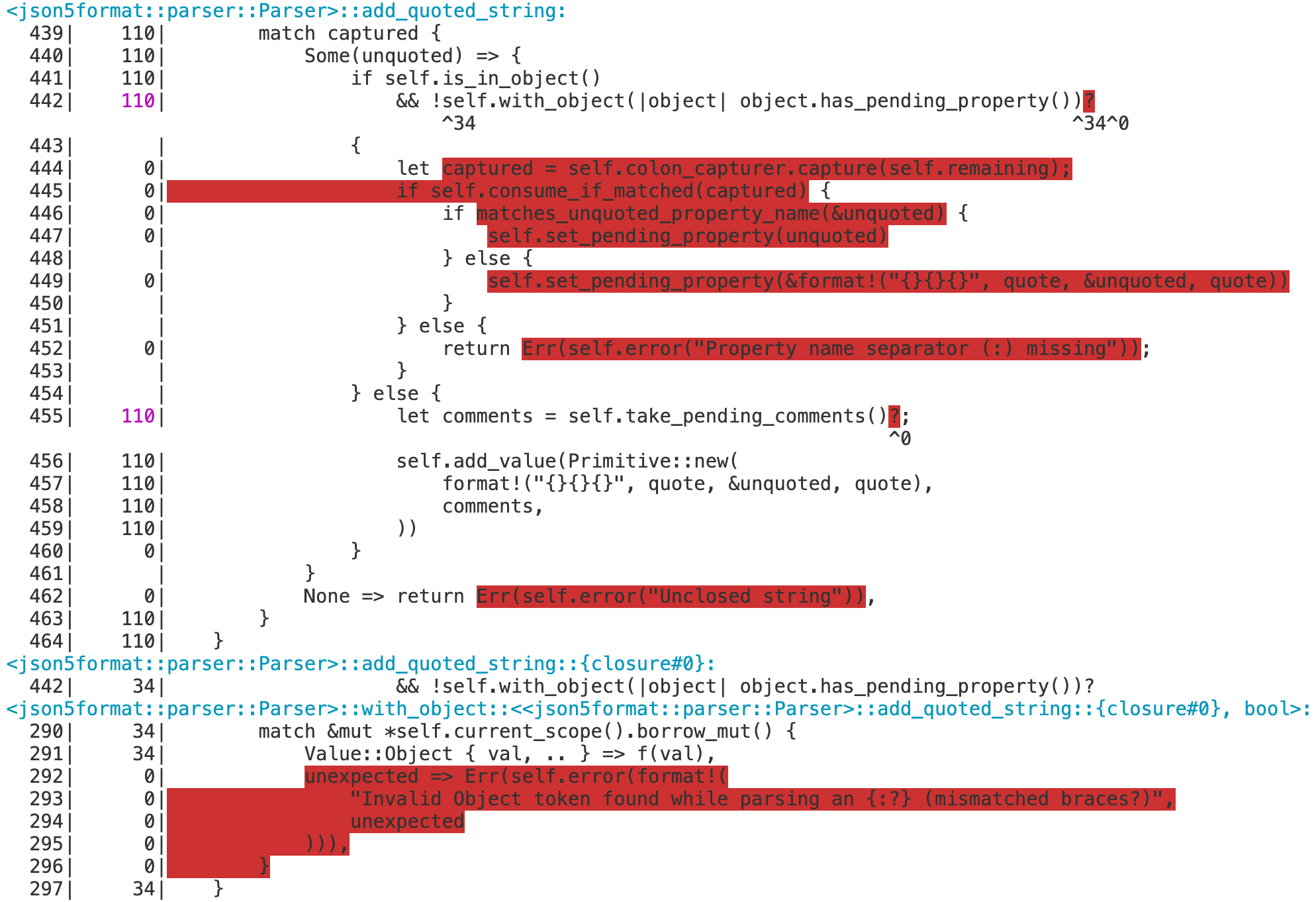
Some of the more notable options in this example include:
--Xdemangler=rustfilt
- the command name or path used to demangle Rust symbols (rustfilt
in the example, but this could also be a path to therust-demangler
tool)target/debug/examples/formatjson5
- the instrumented binary (from which to extract the coverage map)--instr-profile=<path-to-file>.profdata
- the location of the.profdata
file created byllvm-profdata merge
(from the.profraw
file generated by the instrumented binary)--name=<exact-function-name>
- to show coverage for a specific function (or, consider using another filter option, such as--name-regex=<pattern>
)
Note: Coverage can also be disabled on an individual function by annotating the function with the
no_coverage
attribute (which requires the feature flag#![feature(no_coverage)]
).
Interpreting reports
There are four statistics tracked in a coverage summary:
- Function coverage is the percentage of functions that have been executed at least once. A function is considered to be executed if any of its instantiations are executed.
- Instantiation coverage is the percentage of function instantiations that have been executed at least once. Generic functions and functions generated from macros are two kinds of functions that may have multiple instantiations.
- Line coverage is the percentage of code lines that have been executed at least once. Only executable lines within function bodies are considered to be code lines.
- Region coverage is the percentage of code regions that have been executed at least once. A code region may span multiple lines: for example, in a large function body with no control flow. In other cases, a single line can contain multiple code regions:
return x || (y && z)
has countable code regions forx
(which may resolve the expression, ifx
istrue
),|| (y && z)
(executed only ifx
wasfalse
), andreturn
(executed in either situation).
Of these four statistics, function coverage is usually the least granular while region coverage is the most granular. The project-wide totals for each statistic are listed in the summary.
Test coverage
A typical use case for coverage analysis is test coverage. Rust's source-based coverage tools can both measure your tests' code coverage as percentage, and pinpoint functions and branches not tested.
The following example (using the json5format
crate, for demonstration purposes) show how to generate and analyze coverage results for all tests in a crate.
Since cargo test
both builds and runs the tests, we set both the additional RUSTFLAGS
, to add the -Z instrument-coverage
flag, and LLVM_PROFILE_FILE
, to set a custom filename for the raw profiling data generated during the test runs. Since there may be more than one test binary, apply %m
in the filename pattern. This generates unique names for each test binary. (Otherwise, each executed test binary would overwrite the coverage results from the previous binary.)
$ RUSTFLAGS="-Z instrument-coverage" \
LLVM_PROFILE_FILE="json5format-%m.profraw" \
cargo test --tests
Make note of the test binary file paths, displayed after the word "Running
" in the test output:
...
Compiling json5format v0.1.3 ($HOME/json5format)
Finished test [unoptimized + debuginfo] target(s) in 14.60s
Running target/debug/deps/json5format-fececd4653271682
running 25 tests
...
test result: ok. 25 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out
Running target/debug/deps/lib-30768f9c53506dc5
running 31 tests
...
test result: ok. 31 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out
You should have one or more .profraw
files now, one for each test binary. Run the profdata
tool to merge them:
$ cargo profdata -- merge \
-sparse json5format-*.profraw -o json5format.profdata
Then run the cov
tool, with the profdata
file and all test binaries:
$ cargo cov -- report \
--use-color --ignore-filename-regex='/.cargo/registry' \
--instr-profile=json5format.profdata \
--object target/debug/deps/lib-30768f9c53506dc5 \
--object target/debug/deps/json5format-fececd4653271682
$ cargo cov -- show \
--use-color --ignore-filename-regex='/.cargo/registry' \
--instr-profile=json5format.profdata \
--object target/debug/deps/lib-30768f9c53506dc5 \
--object target/debug/deps/json5format-fececd4653271682 \
--show-instantiations --show-line-counts-or-regions \
--Xdemangler=rustfilt | less -R
Note: The command line option
--ignore-filename-regex=/.cargo/registry
, which excludes the sources for dependencies from the coverage results._
Tips for listing the binaries automatically
For bash
users, one suggested way to automatically complete the cov
command with the list of binaries is with a command like:
$ cargo cov -- report \
$( \
for file in \
$( \
RUSTFLAGS="-Z instrument-coverage" \
cargo test --tests --no-run --message-format=json \
| jq -r "select(.profile.test == true) | .filenames[]" \
| grep -v dSYM - \
); \
do \
printf "%s %s " -object $file; \
done \
) \
--instr-profile=json5format.profdata --summary-only # and/or other options
Adding --no-run --message-format=json
to the same cargo test
command used to run
the tests (including the same environment variables and flags) generates output in a JSON
format that jq
can easily query.
The printf
command takes this list and generates the --object <binary>
arguments
for each listed test binary.
Including doc tests
The previous examples run cargo test
with --tests
, which excludes doc tests.1
To include doc tests in the coverage results, drop the --tests
flag, and apply the
-Z instrument-coverage
flag, and some doc-test-specific options in the
RUSTDOCFLAGS
environment variable. (The cargo profdata
command does not change.)
$ RUSTFLAGS="-Z instrument-coverage" \
RUSTDOCFLAGS="-Z instrument-coverage -Z unstable-options --persist-doctests target/debug/doctestbins" \
LLVM_PROFILE_FILE="json5format-%m.profraw" \
cargo test
$ cargo profdata -- merge \
-sparse json5format-*.profraw -o json5format.profdata
The -Z unstable-options --persist-doctests
flag is required, to save the test binaries
(with their coverage maps) for llvm-cov
.
$ cargo cov -- report \
$( \
for file in \
$( \
RUSTFLAGS="-Z instrument-coverage" \
RUSTDOCFLAGS="-Z instrument-coverage -Z unstable-options --persist-doctests target/debug/doctestbins" \
cargo test --no-run --message-format=json \
| jq -r "select(.profile.test == true) | .filenames[]" \
| grep -v dSYM - \
) \
target/debug/doctestbins/*/rust_out; \
do \
[[ -x $file ]] && printf "%s %s " -object $file; \
done \
) \
--instr-profile=json5format.profdata --summary-only # and/or other options
Note: The differences in this
cargo cov
command, compared with the version without doc tests, include:
- The
cargo test ... --no-run
command is updated with the same environment variables and flags used to build the tests, including the doc tests. (LLVM_PROFILE_FILE
is only used when running the tests.) - The file glob pattern
target/debug/doctestbins/*/rust_out
adds therust_out
binaries generated for doc tests (note, however, that somerust_out
files may not be executable binaries). [[ -x $file ]] &&
filters the files passed on to theprintf
, to include only executable binaries.
There is ongoing work to resolve a known issue
[(#79417)](https://github.com/rust-lang/rust/issues/79417) that doc test coverage
generates incorrect source line numbers in `llvm-cov show` results.
-Z instrument-coverage=<options>
-Z instrument-coverage=all
: Instrument all functions, including unused functions and unused generics. (This is the same as-Z instrument-coverage
, with no value.)-Z instrument-coverage=except-unused-generics
: Instrument all functions except unused generics.-Z instrument-coverage=except-unused-functions
: Instrument only used (called) functions and instantiated generic functions.-Z instrument-coverage=off
: Do not instrument any functions. (This is the same as simply not including the-Z instrument-coverage
option.)
Other references
Rust's implementation and workflow for source-based code coverage is based on the same library and tools used to implement source-based code coverage in Clang. (This document is partially based on the Clang guide.)
move_size_limit
The -Zmove-size-limit=N
compiler flag enables large_assignments
lints which
will warn when moving objects whose size exceeds N
bytes.
Lint warns only about moves in functions that participate in code generation.
Consequently it will be ineffective for compiler invocatation that emit
metadata only, i.e., cargo check
like workflows.
profile
The tracking issue for this feature is: #42524.
This feature allows the generation of code coverage reports.
Set the -Zprofile
compiler flag in order to enable gcov profiling.
For example:
cargo new testgcov --bin
cd testgcov
export RUSTFLAGS="-Zprofile -Ccodegen-units=1 -Copt-level=0 -Clink-dead-code -Coverflow-checks=off -Zpanic_abort_tests -Cpanic=abort"
export CARGO_INCREMENTAL=0
cargo build
cargo run
Once you've built and run your program, files with the gcno
(after build) and gcda
(after execution) extensions will be created.
You can parse them with llvm-cov gcov or grcov.
Please note that RUSTFLAGS
by default applies to everything that cargo builds and runs during a build!
When the --target
flag is explicitly passed to cargo, the RUSTFLAGS
no longer apply to build scripts and procedural macros.
For more fine-grained control consider passing a RUSTC_WRAPPER
program to cargo that only adds the profiling flags to
rustc for the specific crates you want to profile.
`profile-sample-use
-Zprofile-sample-use=code.prof
directs rustc
to use the profile
code.prof
as a source for Automatic Feedback Directed Optimization (AFDO).
See the documentation of -Zdebug-info-for-profiling
for more information
on using AFDO.
remap-cwd-prefix
The tracking issue for this feature is: #87325.
This flag will rewrite absolute paths under the current working directory, replacing the current working directory prefix with a specified value.
The given value may be absolute or relative, or empty. This switch takes
precidence over --remap-path-prefix
in case they would both match a given
path.
This flag helps to produce deterministic output, by removing the current working directory from build output, while allowing the command line to be universally reproducible, such that the same execution will work on all machines, regardless of build environment.
Example
# This would produce an absolute path to main.rs in build outputs of
# "./main.rs".
rustc -Z remap-cwd-prefix=. main.rs
report-time
The tracking issue for this feature is: #64888
The report-time
feature adds a possibility to report execution time of the
tests generated via libtest
.
This is unstable feature, so you have to provide -Zunstable-options
to get
this feature working.
Sample usage command:
./test_executable -Zunstable-options --report-time
Available options:
--report-time [plain|colored]
Show execution time of each test. Available values:
plain = do not colorize the execution time (default);
colored = colorize output according to the `color`
parameter value;
Threshold values for colorized output can be
configured via
`RUST_TEST_TIME_UNIT`, `RUST_TEST_TIME_INTEGRATION`
and
`RUST_TEST_TIME_DOCTEST` environment variables.
Expected format of environment variable is
`VARIABLE=WARN_TIME,CRITICAL_TIME`.
Not available for --format=terse
--ensure-time
Treat excess of the test execution time limit as
error.
Threshold values for this option can be configured via
`RUST_TEST_TIME_UNIT`, `RUST_TEST_TIME_INTEGRATION`
and
`RUST_TEST_TIME_DOCTEST` environment variables.
Expected format of environment variable is
`VARIABLE=WARN_TIME,CRITICAL_TIME`.
`CRITICAL_TIME` here means the limit that should not be
exceeded by test.
Example of the environment variable format:
RUST_TEST_TIME_UNIT=100,200
where 100 stands for warn time, and 200 stands for critical time.
Examples
cargo test --tests -- -Zunstable-options --report-time
Finished dev [unoptimized + debuginfo] target(s) in 0.02s
Running target/debug/deps/example-27fb188025bec02c
running 3 tests
test tests::unit_test_quick ... ok <0.000s>
test tests::unit_test_warn ... ok <0.055s>
test tests::unit_test_critical ... ok <0.110s>
test result: ok. 3 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out
Running target/debug/deps/tests-cedb06f6526d15d9
running 3 tests
test unit_test_quick ... ok <0.000s>
test unit_test_warn ... ok <0.550s>
test unit_test_critical ... ok <1.100s>
test result: ok. 3 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out
sanitizer
The tracking issue for this feature is: #39699.
This feature allows for use of one of following sanitizers:
- AddressSanitizer a fast memory error detector.
- HWAddressSanitizer a memory error detector similar to AddressSanitizer, but based on partial hardware assistance.
- LeakSanitizer a run-time memory leak detector.
- MemorySanitizer a detector of uninitialized reads.
- ThreadSanitizer a fast data race detector.
To enable a sanitizer compile with -Zsanitizer=address
,
-Zsanitizer=hwaddress
, -Zsanitizer=leak
, -Zsanitizer=memory
or
-Zsanitizer=thread
.
AddressSanitizer
AddressSanitizer is a memory error detector. It can detect the following types of bugs:
- Out of bound accesses to heap, stack and globals
- Use after free
- Use after return (runtime flag
ASAN_OPTIONS=detect_stack_use_after_return=1
) - Use after scope
- Double-free, invalid free
- Memory leaks
The memory leak detection is enabled by default on Linux, and can be enabled
with runtime flag ASAN_OPTIONS=detect_leaks=1
on macOS.
AddressSanitizer is supported on the following targets:
aarch64-apple-darwin
aarch64-fuchsia
aarch64-unknown-linux-gnu
x86_64-apple-darwin
x86_64-fuchsia
x86_64-unknown-freebsd
x86_64-unknown-linux-gnu
AddressSanitizer works with non-instrumented code although it will impede its ability to detect some bugs. It is not expected to produce false positive reports.
Examples
Stack buffer overflow:
fn main() { let xs = [0, 1, 2, 3]; let _y = unsafe { *xs.as_ptr().offset(4) }; }
$ export RUSTFLAGS=-Zsanitizer=address RUSTDOCFLAGS=-Zsanitizer=address
$ cargo run -Zbuild-std --target x86_64-unknown-linux-gnu
==37882==ERROR: AddressSanitizer: stack-buffer-overflow on address 0x7ffe400e6250 at pc 0x5609a841fb20 bp 0x7ffe400e6210 sp 0x7ffe400e6208
READ of size 4 at 0x7ffe400e6250 thread T0
#0 0x5609a841fb1f in example::main::h628ffc6626ed85b2 /.../src/main.rs:3:23
...
Address 0x7ffe400e6250 is located in stack of thread T0 at offset 48 in frame
#0 0x5609a841f8af in example::main::h628ffc6626ed85b2 /.../src/main.rs:1
This frame has 1 object(s):
[32, 48) 'xs' (line 2) <== Memory access at offset 48 overflows this variable
HINT: this may be a false positive if your program uses some custom stack unwind mechanism, swapcontext or vfork
(longjmp and C++ exceptions *are* supported)
SUMMARY: AddressSanitizer: stack-buffer-overflow /.../src/main.rs:3:23 in example::main::h628ffc6626ed85b2
Shadow bytes around the buggy address:
0x100048014bf0: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0x100048014c00: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0x100048014c10: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0x100048014c20: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0x100048014c30: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
=>0x100048014c40: 00 00 00 00 f1 f1 f1 f1 00 00[f3]f3 00 00 00 00
0x100048014c50: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0x100048014c60: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0x100048014c70: f1 f1 f1 f1 00 00 f3 f3 00 00 00 00 00 00 00 00
0x100048014c80: 00 00 00 00 00 00 00 00 00 00 00 00 f1 f1 f1 f1
0x100048014c90: 00 00 f3 f3 00 00 00 00 00 00 00 00 00 00 00 00
Shadow byte legend (one shadow byte represents 8 application bytes):
Addressable: 00
Partially addressable: 01 02 03 04 05 06 07
Heap left redzone: fa
Freed heap region: fd
Stack left redzone: f1
Stack mid redzone: f2
Stack right redzone: f3
Stack after return: f5
Stack use after scope: f8
Global redzone: f9
Global init order: f6
Poisoned by user: f7
Container overflow: fc
Array cookie: ac
Intra object redzone: bb
ASan internal: fe
Left alloca redzone: ca
Right alloca redzone: cb
Shadow gap: cc
==37882==ABORTING
Use of a stack object after its scope has already ended:
static mut P: *mut usize = std::ptr::null_mut(); fn main() { unsafe { { let mut x = 0; P = &mut x; } std::ptr::write_volatile(P, 123); } }
$ export RUSTFLAGS=-Zsanitizer=address RUSTDOCFLAGS=-Zsanitizer=address
$ cargo run -Zbuild-std --target x86_64-unknown-linux-gnu
=================================================================
==39249==ERROR: AddressSanitizer: stack-use-after-scope on address 0x7ffc7ed3e1a0 at pc 0x55c98b262a8e bp 0x7ffc7ed3e050 sp 0x7ffc7ed3e048
WRITE of size 8 at 0x7ffc7ed3e1a0 thread T0
#0 0x55c98b262a8d in core::ptr::write_volatile::he21f1df5a82f329a /.../src/rust/src/libcore/ptr/mod.rs:1048:5
#1 0x55c98b262cd2 in example::main::h628ffc6626ed85b2 /.../src/main.rs:9:9
...
Address 0x7ffc7ed3e1a0 is located in stack of thread T0 at offset 32 in frame
#0 0x55c98b262bdf in example::main::h628ffc6626ed85b2 /.../src/main.rs:3
This frame has 1 object(s):
[32, 40) 'x' (line 6) <== Memory access at offset 32 is inside this variable
HINT: this may be a false positive if your program uses some custom stack unwind mechanism, swapcontext or vfork
(longjmp and C++ exceptions *are* supported)
SUMMARY: AddressSanitizer: stack-use-after-scope /.../src/rust/src/libcore/ptr/mod.rs:1048:5 in core::ptr::write_volatile::he21f1df5a82f329a
Shadow bytes around the buggy address:
0x10000fd9fbe0: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0x10000fd9fbf0: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0x10000fd9fc00: 00 00 00 00 00 00 00 00 00 00 00 00 f1 f1 f1 f1
0x10000fd9fc10: f8 f8 f3 f3 00 00 00 00 00 00 00 00 00 00 00 00
0x10000fd9fc20: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
=>0x10000fd9fc30: f1 f1 f1 f1[f8]f3 f3 f3 00 00 00 00 00 00 00 00
0x10000fd9fc40: 00 00 00 00 00 00 00 00 00 00 00 00 f1 f1 f1 f1
0x10000fd9fc50: 00 00 f3 f3 00 00 00 00 00 00 00 00 00 00 00 00
0x10000fd9fc60: 00 00 00 00 00 00 00 00 f1 f1 f1 f1 00 00 f3 f3
0x10000fd9fc70: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0x10000fd9fc80: 00 00 00 00 f1 f1 f1 f1 00 00 f3 f3 00 00 00 00
Shadow byte legend (one shadow byte represents 8 application bytes):
Addressable: 00
Partially addressable: 01 02 03 04 05 06 07
Heap left redzone: fa
Freed heap region: fd
Stack left redzone: f1
Stack mid redzone: f2
Stack right redzone: f3
Stack after return: f5
Stack use after scope: f8
Global redzone: f9
Global init order: f6
Poisoned by user: f7
Container overflow: fc
Array cookie: ac
Intra object redzone: bb
ASan internal: fe
Left alloca redzone: ca
Right alloca redzone: cb
Shadow gap: cc
==39249==ABORTING
HWAddressSanitizer
HWAddressSanitizer is a newer variant of AddressSanitizer that consumes much less memory.
HWAddressSanitizer is supported on the following targets:
aarch64-linux-android
aarch64-unknown-linux-gnu
HWAddressSanitizer requires tagged-globals
target feature to instrument
globals. To enable this target feature compile with -C target-feature=+tagged-globals
Example
Heap buffer overflow:
fn main() { let xs = vec![0, 1, 2, 3]; let _y = unsafe { *xs.as_ptr().offset(4) }; }
$ rustc main.rs -Zsanitizer=hwaddress -C target-feature=+tagged-globals -C
linker=aarch64-linux-gnu-gcc -C link-arg=-fuse-ld=lld --target
aarch64-unknown-linux-gnu
$ ./main
==241==ERROR: HWAddressSanitizer: tag-mismatch on address 0xefdeffff0050 at pc 0xaaaae0ae4a98
READ of size 4 at 0xefdeffff0050 tags: 2c/00 (ptr/mem) in thread T0
#0 0xaaaae0ae4a94 (/.../main+0x54a94)
...
[0xefdeffff0040,0xefdeffff0060) is a small allocated heap chunk; size: 32 offset: 16
0xefdeffff0050 is located 0 bytes to the right of 16-byte region [0xefdeffff0040,0xefdeffff0050)
allocated here:
#0 0xaaaae0acb80c (/.../main+0x3b80c)
...
Thread: T0 0xeffe00002000 stack: [0xffffc28ad000,0xffffc30ad000) sz: 8388608 tls: [0xffffaa10a020,0xffffaa10a7d0)
Memory tags around the buggy address (one tag corresponds to 16 bytes):
0xfefcefffef80: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0xfefcefffef90: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0xfefcefffefa0: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0xfefcefffefb0: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0xfefcefffefc0: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0xfefcefffefd0: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0xfefcefffefe0: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0xfefcefffeff0: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
=>0xfefceffff000: d7 d7 05 00 2c [00] 00 00 00 00 00 00 00 00 00 00
0xfefceffff010: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0xfefceffff020: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0xfefceffff030: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0xfefceffff040: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0xfefceffff050: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0xfefceffff060: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0xfefceffff070: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
0xfefceffff080: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
Tags for short granules around the buggy address (one tag corresponds to 16 bytes):
0xfefcefffeff0: .. .. .. .. .. .. .. .. .. .. .. .. .. .. .. ..
=>0xfefceffff000: .. .. 8c .. .. [..] .. .. .. .. .. .. .. .. .. ..
0xfefceffff010: .. .. .. .. .. .. .. .. .. .. .. .. .. .. .. ..
See https://clang.llvm.org/docs/HardwareAssistedAddressSanitizerDesign.html#short-granules for a description of short granule tags
Registers where the failure occurred (pc 0xaaaae0ae4a98):
x0 2c00efdeffff0050 x1 0000000000000004 x2 0000000000000004 x3 0000000000000000
x4 0000fffefc30ac37 x5 000000000000005d x6 00000ffffc30ac37 x7 0000efff00000000
x8 2c00efdeffff0050 x9 0200efff00000000 x10 0000000000000000 x11 0200efff00000000
x12 0200effe00000310 x13 0200effe00000310 x14 0000000000000008 x15 5d00ffffc30ac360
x16 0000aaaae0ad062c x17 0000000000000003 x18 0000000000000001 x19 0000ffffc30ac658
x20 4e00ffffc30ac6e0 x21 0000aaaae0ac5e10 x22 0000000000000000 x23 0000000000000000
x24 0000000000000000 x25 0000000000000000 x26 0000000000000000 x27 0000000000000000
x28 0000000000000000 x29 0000ffffc30ac5a0 x30 0000aaaae0ae4a98
SUMMARY: HWAddressSanitizer: tag-mismatch (/.../main+0x54a94)
LeakSanitizer
LeakSanitizer is run-time memory leak detector.
LeakSanitizer is supported on the following targets:
aarch64-apple-darwin
aarch64-unknown-linux-gnu
x86_64-apple-darwin
x86_64-unknown-linux-gnu
MemorySanitizer
MemorySanitizer is detector of uninitialized reads.
MemorySanitizer is supported on the following targets:
aarch64-unknown-linux-gnu
x86_64-unknown-freebsd
x86_64-unknown-linux-gnu
MemorySanitizer requires all program code to be instrumented. C/C++ dependencies
need to be recompiled using Clang with -fsanitize=memory
option. Failing to
achieve that will result in false positive reports.
Example
Detecting the use of uninitialized memory. The -Zbuild-std
flag rebuilds and
instruments the standard library, and is strictly necessary for the correct
operation of the tool. The -Zsanitizer-memory-track-origins
enables tracking
of the origins of uninitialized memory:
use std::mem::MaybeUninit; fn main() { unsafe { let a = MaybeUninit::<[usize; 4]>::uninit(); let a = a.assume_init(); println!("{}", a[2]); } }
$ export \
RUSTFLAGS='-Zsanitizer=memory -Zsanitizer-memory-track-origins' \
RUSTDOCFLAGS='-Zsanitizer=memory -Zsanitizer-memory-track-origins'
$ cargo clean
$ cargo run -Zbuild-std --target x86_64-unknown-linux-gnu
==9416==WARNING: MemorySanitizer: use-of-uninitialized-value
#0 0x560c04f7488a in core::fmt::num::imp::fmt_u64::haa293b0b098501ca $RUST/build/x86_64-unknown-linux-gnu/stage1/lib/rustlib/src/rust/src/libcore/fmt/num.rs:202:16
...
Uninitialized value was stored to memory at
#0 0x560c04ae898a in __msan_memcpy.part.0 $RUST/src/llvm-project/compiler-rt/lib/msan/msan_interceptors.cc:1558:3
#1 0x560c04b2bf88 in memory::main::hd2333c1899d997f5 $CWD/src/main.rs:6:16
Uninitialized value was created by an allocation of 'a' in the stack frame of function '_ZN6memory4main17hd2333c1899d997f5E'
#0 0x560c04b2bc50 in memory::main::hd2333c1899d997f5 $CWD/src/main.rs:3
ThreadSanitizer
ThreadSanitizer is a data race detection tool. It is supported on the following targets:
aarch64-apple-darwin
aarch64-unknown-linux-gnu
x86_64-apple-darwin
x86_64-unknown-freebsd
x86_64-unknown-linux-gnu
To work correctly ThreadSanitizer needs to be "aware" of all synchronization
operations in a program. It generally achieves that through combination of
library interception (for example synchronization performed through
pthread_mutex_lock
/ pthread_mutex_unlock
) and compile time instrumentation
(e.g. atomic operations). Using it without instrumenting all the program code
can lead to false positive reports.
ThreadSanitizer does not support atomic fences std::sync::atomic::fence
,
nor synchronization performed using inline assembly code.
Example
static mut A: usize = 0; fn main() { let t = std::thread::spawn(|| { unsafe { A += 1 }; }); unsafe { A += 1 }; t.join().unwrap(); }
$ export RUSTFLAGS=-Zsanitizer=thread RUSTDOCFLAGS=-Zsanitizer=thread
$ cargo run -Zbuild-std --target x86_64-unknown-linux-gnu
==================
WARNING: ThreadSanitizer: data race (pid=10574)
Read of size 8 at 0x5632dfe3d030 by thread T1:
#0 example::main::_$u7b$$u7b$closure$u7d$$u7d$::h23f64b0b2f8c9484 ../src/main.rs:5:18 (example+0x86cec)
...
Previous write of size 8 at 0x5632dfe3d030 by main thread:
#0 example::main::h628ffc6626ed85b2 /.../src/main.rs:7:14 (example+0x868c8)
...
#11 main <null> (example+0x86a1a)
Location is global 'example::A::h43ac149ddf992709' of size 8 at 0x5632dfe3d030 (example+0x000000bd9030)
Instrumentation of external dependencies and std
The sanitizers to varying degrees work correctly with partially instrumented code. On the one extreme is LeakSanitizer that doesn't use any compile time instrumentation, on the other is MemorySanitizer that requires that all program code to be instrumented (failing to achieve that will inevitably result in false positives).
It is strongly recommended to combine sanitizers with recompiled and
instrumented standard library, for example using cargo -Zbuild-std
functionality.
Build scripts and procedural macros
Use of sanitizers together with build scripts and procedural macros is technically possible, but in almost all cases it would be best avoided. This is especially true for procedural macros which would require an instrumented version of rustc.
In more practical terms when using cargo always remember to pass --target
flag, so that rustflags will not be applied to build scripts and procedural
macros.
Symbolizing the Reports
Sanitizers produce symbolized stacktraces when llvm-symbolizer binary is in PATH
.
Additional Information
- Sanitizers project page
- AddressSanitizer in Clang
- HWAddressSanitizer in Clang
- LeakSanitizer in Clang
- MemorySanitizer in Clang
- ThreadSanitizer in Clang
self-profile
The -Zself-profile
compiler flag enables rustc's internal profiler.
When enabled, the compiler will output three binary files in the specified directory (or the current working directory if no directory is specified).
These files can be analyzed by using the tools in the measureme
repository.
To control the data recorded in the trace files, use the -Zself-profile-events
flag.
For example:
First, run a compilation session and provide the -Zself-profile
flag:
$ rustc --crate-name foo -Zself-profile
This will generate three files in the working directory such as:
foo-1234.events
foo-1234.string_data
foo-1234.string_index
Where foo
is the name of the crate and 1234
is the process id of the rustc process.
To get a summary of where the compiler is spending its time:
$ ../measureme/target/release/summarize summarize foo-1234
To generate a flamegraph of the same data:
$ ../measureme/target/release/inferno foo-1234
To dump the event data in a Chromium-profiler compatible format:
$ ../measureme/target/release/crox foo-1234
For more information, consult the measureme
documentation.
self-profile-events
The -Zself-profile-events
compiler flag controls what events are recorded by the self-profiler when it is enabled via the -Zself-profile
flag.
This flag takes a comma delimited list of event types to record.
For example:
$ rustc -Zself-profile -Zself-profile-events=default,args
Event types
-
query-provider
- Traces each query used internally by the compiler.
-
generic-activity
- Traces other parts of the compiler not covered by the query system.
-
query-cache-hit
- Adds tracing information that records when the in-memory query cache is "hit" and does not need to re-execute a query which has been cached.
- Disabled by default because this significantly increases the trace file size.
-
query-blocked
- Tracks time that a query tries to run but is blocked waiting on another thread executing the same query to finish executing.
- Query blocking only occurs when the compiler is built with parallel mode support.
-
incr-cache-load
- Tracks time that is spent loading and deserializing query results from the incremental compilation on-disk cache.
-
query-keys
- Adds a serialized representation of each query's query key to the tracing data.
- Disabled by default because this significantly increases the trace file size.
-
function-args
- Adds additional tracing data to some
generic-activity
events. - Disabled by default for parity with
query-keys
.
- Adds additional tracing data to some
-
llvm
- Adds tracing information about LLVM passes and codegeneration.
- Disabled by default because this only works when
-Znew-llvm-pass-manager
is enabled.
Event synonyms
-
none
- Disables all events. Equivalent to the self-profiler being disabled.
-
default
- The default set of events which stikes a balance between providing detailed tracing data and adding additional overhead to the compilation.
-
args
- Equivalent to
query-keys
andfunction-args
.
- Equivalent to
-
all
- Enables all events.
Examples
Enable the profiler and capture the default set of events (both invocations are equivalent):
$ rustc -Zself-profile
$ rustc -Zself-profile -Zself-profile-events=default
Enable the profiler and capture the default events and their arguments:
$ rustc -Zself-profile -Zself-profile-events=default,args
source-based-code-coverage
See compiler flag -Z instrument-coverage
.
src-hash-algorithm
The tracking issue for this feature is: #70401.
The -Z src-hash-algorithm
compiler flag controls which algorithm is used when hashing each source file. The hash is stored in the debug info and can be used by a debugger to verify the source code matches the executable.
Supported hash algorithms are: md5
, sha1
, and sha256
. Note that not all hash algorithms are supported by all debug info formats.
By default, the compiler chooses the hash algorithm based on the target specification.
strip
The tracking issue for this feature is: #72110.
Option -Z strip=val
controls stripping of debuginfo and similar auxiliary data from binaries
during linking.
Supported values for this option are:
none
- debuginfo and symbols (if they exist) are copied to the produced binary or separate files depending on the target (e.g..pdb
files in case of MSVC).debuginfo
- debuginfo sections and debuginfo symbols from the symbol table section are stripped at link time and are not copied to the produced binary or separate files.symbols
- same asdebuginfo
, but the rest of the symbol table section is stripped as well if the linker supports it.
tls_model
The tracking issue for this feature is: None.
Option -Z tls-model
controls TLS model used to
generate code for accessing #[thread_local]
static
items.
Supported values for this option are:
global-dynamic
- General Dynamic TLS Model (alternatively called Global Dynamic) is the most general option usable in all circumstances, even if the TLS data is defined in a shared library loaded at runtime and is accessed from code outside of that library. This is the default for most targets.local-dynamic
- model usable if the TLS data is only accessed from the shared library or executable it is defined in. The TLS data may be in a library loaded after startup (viadlopen
).initial-exec
- model usable if the TLS data is defined in the executable or in a shared library loaded at program startup. The TLS data must not be in a library loaded after startup (viadlopen
).local-exec
- model usable only if the TLS data is defined directly in the executable, but not in a shared library, and is accessed only from that executable.
rustc
and LLVM may use a more optimized model than specified if they know that we are producing
an executable rather than a library, or that the static
item is private enough.
unsound-mir-opts
The -Zunsound-mir-opts
compiler flag enables MIR optimization passes which can cause unsound behavior.
This flag should only be used by MIR optimization tests in the rustc test suite.
Language features
aarch64_target_feature
The tracking issue for this feature is: #44839
abi_amdgpu_kernel
The tracking issue for this feature is: #51575
abi_avr_interrupt
The tracking issue for this feature is: #69664
abi_c_cmse_nonsecure_call
The tracking issue for this feature is: #81391
The TrustZone-M
feature is available
for targets with the Armv8-M architecture profile (thumbv8m
in their target
name).
LLVM, the Rust compiler and the linker are providing
support for the
TrustZone-M feature.
One of the things provided, with this unstable feature, is the
C-cmse-nonsecure-call
function ABI. This ABI is used on function pointers to
non-secure code to mark a non-secure function call (see section
5.5 for details).
With this ABI, the compiler will do the following to perform the call:
- save registers needed after the call to Secure memory
- clear all registers that might contain confidential information
- clear the Least Significant Bit of the function address
- branches using the BLXNS instruction
To avoid using the non-secure stack, the compiler will constrain the number and type of parameters/return value.
The extern "C-cmse-nonsecure-call"
ABI is otherwise equivalent to the
extern "C"
ABI.
#![no_std]
#![feature(abi_c_cmse_nonsecure_call)]
#[no_mangle]
pub fn call_nonsecure_function(addr: usize) -> u32 {
let non_secure_function =
unsafe { core::mem::transmute::<usize, extern "C-cmse-nonsecure-call" fn() -> u32>(addr) };
non_secure_function()
}
$ rustc --emit asm --crate-type lib --target thumbv8m.main-none-eabi function.rs
call_nonsecure_function:
.fnstart
.save {r7, lr}
push {r7, lr}
.setfp r7, sp
mov r7, sp
.pad #16
sub sp, #16
str r0, [sp, #12]
ldr r0, [sp, #12]
str r0, [sp, #8]
b .LBB0_1
.LBB0_1:
ldr r0, [sp, #8]
push.w {r4, r5, r6, r7, r8, r9, r10, r11}
bic r0, r0, #1
mov r1, r0
mov r2, r0
mov r3, r0
mov r4, r0
mov r5, r0
mov r6, r0
mov r7, r0
mov r8, r0
mov r9, r0
mov r10, r0
mov r11, r0
mov r12, r0
msr apsr_nzcvq, r0
blxns r0
pop.w {r4, r5, r6, r7, r8, r9, r10, r11}
str r0, [sp, #4]
b .LBB0_2
.LBB0_2:
ldr r0, [sp, #4]
add sp, #16
pop {r7, pc}
abi_efiapi
The tracking issue for this feature is: #65815
abi_msp430_interrupt
The tracking issue for this feature is: #38487
In the MSP430 architecture, interrupt handlers have a special calling
convention. You can use the "msp430-interrupt"
ABI to make the compiler apply
the right calling convention to the interrupt handlers you define.
#![feature(abi_msp430_interrupt)]
#![no_std]
// Place the interrupt handler at the appropriate memory address
// (Alternatively, you can use `#[used]` and remove `pub` and `#[no_mangle]`)
#[link_section = "__interrupt_vector_10"]
#[no_mangle]
pub static TIM0_VECTOR: extern "msp430-interrupt" fn() = tim0;
// The interrupt handler
extern "msp430-interrupt" fn tim0() {
// ..
}
$ msp430-elf-objdump -CD ./target/msp430/release/app
Disassembly of section __interrupt_vector_10:
0000fff2 <TIM0_VECTOR>:
fff2: 00 c0 interrupt service routine at 0xc000
Disassembly of section .text:
0000c000 <int::tim0>:
c000: 00 13 reti
abi_ptx
The tracking issue for this feature is: #38788
When emitting PTX code, all vanilla Rust functions (fn
) get translated to
"device" functions. These functions are not callable from the host via the
CUDA API so a crate with only device functions is not too useful!
OTOH, "global" functions can be called by the host; you can think of them
as the real public API of your crate. To produce a global function use the
"ptx-kernel"
ABI.
#![feature(abi_ptx)]
#![no_std]
pub unsafe extern "ptx-kernel" fn global_function() {
device_function();
}
pub fn device_function() {
// ..
}
$ xargo rustc --target nvptx64-nvidia-cuda --release -- --emit=asm
$ cat $(find -name '*.s')
//
// Generated by LLVM NVPTX Back-End
//
.version 3.2
.target sm_20
.address_size 64
// .globl _ZN6kernel15global_function17h46111ebe6516b382E
.visible .entry _ZN6kernel15global_function17h46111ebe6516b382E()
{
ret;
}
// .globl _ZN6kernel15device_function17hd6a0e4993bbf3f78E
.visible .func _ZN6kernel15device_function17hd6a0e4993bbf3f78E()
{
ret;
}
abi_thiscall
The tracking issue for this feature is: #42202
The MSVC ABI on x86 Windows uses the thiscall
calling convention for C++
instance methods by default; it is identical to the usual (C) calling
convention on x86 Windows except that the first parameter of the method,
the this
pointer, is passed in the ECX register.
abi_unadjusted
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
abi_vectorcall
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
abi_x86_interrupt
The tracking issue for this feature is: #40180
adt_const_params
The tracking issue for this feature is: #44580
adx_target_feature
The tracking issue for this feature is: #44839
alloc_error_handler
The tracking issue for this feature is: #51540
allocator_internals
This feature does not have a tracking issue, it is an unstable implementation
detail of the global_allocator
feature not intended for use outside the
compiler.
allow_fail
The tracking issue for this feature is: #46488
allow_internal_unsafe
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
allow_internal_unstable
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
arbitrary_enum_discriminant
The tracking issue for this feature is: #60553
The arbitrary_enum_discriminant
feature permits tuple-like and
struct-like enum variants with #[repr(<int-type>)]
to have explicit discriminants.
Examples
#![allow(unused)] #![feature(arbitrary_enum_discriminant)] fn main() { #[allow(dead_code)] #[repr(u8)] enum Enum { Unit = 3, Tuple(u16) = 2, Struct { a: u8, b: u16, } = 1, } impl Enum { fn tag(&self) -> u8 { unsafe { *(self as *const Self as *const u8) } } } assert_eq!(3, Enum::Unit.tag()); assert_eq!(2, Enum::Tuple(5).tag()); assert_eq!(1, Enum::Struct{a: 7, b: 11}.tag()); }
arbitrary_self_types
The tracking issue for this feature is: #44874
arm_target_feature
The tracking issue for this feature is: #44839
associated_type_bounds
The tracking issue for this feature is: #52662
associated_type_defaults
The tracking issue for this feature is: #29661
async_closure
The tracking issue for this feature is: #62290
auto_traits
The tracking issue for this feature is #13231
The auto_traits
feature gate allows you to define auto traits.
Auto traits, like Send
or Sync
in the standard library, are marker traits
that are automatically implemented for every type, unless the type, or a type it contains,
has explicitly opted out via a negative impl. (Negative impls are separately controlled
by the negative_impls
feature.)
impl !Trait for Type {}
Example:
#![feature(negative_impls)] #![feature(auto_traits)] auto trait Valid {} struct True; struct False; impl !Valid for False {} struct MaybeValid<T>(T); fn must_be_valid<T: Valid>(_t: T) { } fn main() { // works must_be_valid( MaybeValid(True) ); // compiler error - trait bound not satisfied // must_be_valid( MaybeValid(False) ); }
Automatic trait implementations
When a type is declared as an auto trait
, we will automatically
create impls for every struct/enum/union, unless an explicit impl is
provided. These automatic impls contain a where clause for each field
of the form T: AutoTrait
, where T
is the type of the field and
AutoTrait
is the auto trait in question. As an example, consider the
struct List
and the auto trait Send
:
#![allow(unused)] fn main() { struct List<T> { data: T, next: Option<Box<List<T>>>, } }
Presuming that there is no explicit impl of Send
for List
, the
compiler will supply an automatic impl of the form:
#![allow(unused)] fn main() { struct List<T> { data: T, next: Option<Box<List<T>>>, } unsafe impl<T> Send for List<T> where T: Send, // from the field `data` Option<Box<List<T>>>: Send, // from the field `next` { } }
Explicit impls may be either positive or negative. They take the form:
impl<...> AutoTrait for StructName<..> { }
impl<...> !AutoTrait for StructName<..> { }
Coinduction: Auto traits permit cyclic matching
Unlike ordinary trait matching, auto traits are coinductive. This
means, in short, that cycles which occur in trait matching are
considered ok. As an example, consider the recursive struct List
introduced in the previous section. In attempting to determine whether
List: Send
, we would wind up in a cycle: to apply the impl, we must
show that Option<Box<List>>: Send
, which will in turn require
Box<List>: Send
and then finally List: Send
again. Under ordinary
trait matching, this cycle would be an error, but for an auto trait it
is considered a successful match.
Items
Auto traits cannot have any trait items, such as methods or associated types. This ensures that we can generate default implementations.
Supertraits
Auto traits cannot have supertraits. This is for soundness reasons, as the interaction of coinduction with implied bounds is difficult to reconcile.
avx512_target_feature
The tracking issue for this feature is: #44839
box_patterns
The tracking issue for this feature is: #29641
See also box_syntax
Box patterns let you match on Box<T>
s:
#![feature(box_patterns)] fn main() { let b = Some(Box::new(5)); match b { Some(box n) if n < 0 => { println!("Box contains negative number {}", n); }, Some(box n) if n >= 0 => { println!("Box contains non-negative number {}", n); }, None => { println!("No box"); }, _ => unreachable!() } }
box_syntax
The tracking issue for this feature is: #49733
See also box_patterns
Currently the only stable way to create a Box
is via the Box::new
method.
Also it is not possible in stable Rust to destructure a Box
in a match
pattern. The unstable box
keyword can be used to create a Box
. An example
usage would be:
#![feature(box_syntax)] fn main() { let b = box 5; }
bpf_target_feature
The tracking issue for this feature is: #44839
c_unwind
The tracking issue for this feature is: #74990
Introduces four new ABI strings: "C-unwind", "stdcall-unwind", "thiscall-unwind", and "system-unwind". These enable unwinding from other languages (such as C++) into Rust frames and from Rust into other languages.
See RFC 2945 for more information.
c_variadic
The tracking issue for this feature is: #44930
The c_variadic
language feature enables C-variadic functions to be
defined in Rust. The may be called both from within Rust and via FFI.
Examples
#![allow(unused)] #![feature(c_variadic)] fn main() { pub unsafe extern "C" fn add(n: usize, mut args: ...) -> usize { let mut sum = 0; for _ in 0..n { sum += args.arg::<usize>(); } sum } }
capture_disjoint_fields
The tracking issue for this feature is: #53488
cfg_panic
The tracking issue for this feature is: #77443
The cfg_panic
feature makes it possible to execute different code
depending on the panic strategy.
Possible values at the moment are "unwind"
or "abort"
, although
it is possible that new panic strategies may be added to Rust in the
future.
Examples
#![allow(unused)] #![feature(cfg_panic)] fn main() { #[cfg(panic = "unwind")] fn a() { // ... } #[cfg(not(panic = "unwind"))] fn a() { // ... } fn b() { if cfg!(panic = "abort") { // ... } else { // ... } } }
cfg_sanitize
The tracking issue for this feature is: #39699
The cfg_sanitize
feature makes it possible to execute different code
depending on whether a particular sanitizer is enabled or not.
Examples
#![allow(unused)] #![feature(cfg_sanitize)] fn main() { #[cfg(sanitize = "thread")] fn a() { // ... } #[cfg(not(sanitize = "thread"))] fn a() { // ... } fn b() { if cfg!(sanitize = "leak") { // ... } else { // ... } } }
cfg_target_abi
The tracking issue for this feature is: #80970
cfg_target_has_atomic
The tracking issue for this feature is: #32976
cfg_target_thread_local
The tracking issue for this feature is: #29594
cfg_version
The tracking issue for this feature is: #64796
The cfg_version
feature makes it possible to execute different code
depending on the compiler version. It will return true if the compiler
version is greater than or equal to the specified version.
Examples
#![allow(unused)] #![feature(cfg_version)] fn main() { #[cfg(version("1.42"))] // 1.42 and above fn a() { // ... } #[cfg(not(version("1.42")))] // 1.41 and below fn a() { // ... } fn b() { if cfg!(version("1.42")) { // ... } else { // ... } } }
closure_track_caller
The tracking issue for this feature is: #87417
Allows using the #[track_caller]
attribute on closures and generators.
Calls made to the closure or generator will have caller information
available through std::panic::Location::caller()
, just like using
#[track_caller]
on a function.
cmpxchg16b_target_feature
The tracking issue for this feature is: #44839
cmse_nonsecure_entry
The tracking issue for this feature is: #75835
The TrustZone-M
feature is available
for targets with the Armv8-M architecture profile (thumbv8m
in their target
name).
LLVM, the Rust compiler and the linker are providing
support for the
TrustZone-M feature.
One of the things provided, with this unstable feature, is the
cmse_nonsecure_entry
attribute. This attribute marks a Secure function as an
entry function (see section
5.4 for details).
With this attribute, the compiler will do the following:
- add a special symbol on the function which is the
__acle_se_
prefix and the standard function name - constrain the number of parameters to avoid using the Non-Secure stack
- before returning from the function, clear registers that might contain Secure information
- use the
BXNS
instruction to return
Because the stack can not be used to pass parameters, there will be compilation errors if:
- the total size of all parameters is too big (for example more than four 32 bits integers)
- the entry function is not using a C ABI
The special symbol __acle_se_
will be used by the linker to generate a secure
gateway veneer.
#![feature(cmse_nonsecure_entry)]
#[no_mangle]
#[cmse_nonsecure_entry]
pub extern "C" fn entry_function(input: u32) -> u32 {
input + 6
}
$ rustc --emit obj --crate-type lib --target thumbv8m.main-none-eabi function.rs
$ arm-none-eabi-objdump -D function.o
00000000 <entry_function>:
0: b580 push {r7, lr}
2: 466f mov r7, sp
4: b082 sub sp, #8
6: 9001 str r0, [sp, #4]
8: 1d81 adds r1, r0, #6
a: 460a mov r2, r1
c: 4281 cmp r1, r0
e: 9200 str r2, [sp, #0]
10: d30b bcc.n 2a <entry_function+0x2a>
12: e7ff b.n 14 <entry_function+0x14>
14: 9800 ldr r0, [sp, #0]
16: b002 add sp, #8
18: e8bd 4080 ldmia.w sp!, {r7, lr}
1c: 4671 mov r1, lr
1e: 4672 mov r2, lr
20: 4673 mov r3, lr
22: 46f4 mov ip, lr
24: f38e 8800 msr CPSR_f, lr
28: 4774 bxns lr
2a: f240 0000 movw r0, #0
2e: f2c0 0000 movt r0, #0
32: f240 0200 movw r2, #0
36: f2c0 0200 movt r2, #0
3a: 211c movs r1, #28
3c: f7ff fffe bl 0 <_ZN4core9panicking5panic17h5c028258ca2fb3f5E>
40: defe udf #254 ; 0xfe
compiler_builtins
This feature is internal to the Rust compiler and is not intended for general use.
const_async_blocks
The tracking issue for this feature is: #85368
const_eval_limit
The tracking issue for this feature is: #67217
The const_eval_limit
allows someone to limit the evaluation steps the CTFE undertakes to evaluate a const fn
.
const_extern_fn
The tracking issue for this feature is: #64926
const_fn_floating_point_arithmetic
The tracking issue for this feature is: #57241
const_fn_fn_ptr_basics
The tracking issue for this feature is: #57563
const_fn_trait_bound
The tracking issue for this feature is: #57563
const_for
The tracking issue for this feature is: #87575
const_generics_defaults
The tracking issue for this feature is: #44580
const_impl_trait
The tracking issue for this feature is: #77463
const_mut_refs
The tracking issue for this feature is: #57349
const_precise_live_drops
The tracking issue for this feature is: #73255
const_raw_ptr_deref
The tracking issue for this feature is: #51911
const_refs_to_cell
The tracking issue for this feature is: #80384
const_trait_impl
The tracking issue for this feature is: #67792
const_try
The tracking issue for this feature is: #74935
crate_visibility_modifier
The tracking issue for this feature is: #53120
The crate_visibility_modifier
feature allows the crate
keyword to be used
as a visibility modifier synonymous to pub(crate)
, indicating that a type
(function, &c.) is to be visible to the entire enclosing crate, but not to
other crates.
#![allow(unused)] #![feature(crate_visibility_modifier)] fn main() { crate struct Foo { bar: usize, } }
custom_inner_attributes
The tracking issue for this feature is: #54726
custom_test_frameworks
The tracking issue for this feature is: #50297
The custom_test_frameworks
feature allows the use of #[test_case]
and #![test_runner]
.
Any function, const, or static can be annotated with #[test_case]
causing it to be aggregated (like #[test]
)
and be passed to the test runner determined by the #![test_runner]
crate attribute.
#![allow(unused)] #![feature(custom_test_frameworks)] #![test_runner(my_runner)] fn main() { fn my_runner(tests: &[&i32]) { for t in tests { if **t == 0 { println!("PASSED"); } else { println!("FAILED"); } } } #[test_case] const WILL_PASS: i32 = 0; #[test_case] const WILL_FAIL: i32 = 4; }
decl_macro
The tracking issue for this feature is: #39412
default_alloc_error_handler
The tracking issue for this feature is: #66741
default_type_parameter_fallback
The tracking issue for this feature is: #27336
derive_default_enum
The tracking issue for this feature is: #86985
destructuring_assignment
The tracking issue for this feature is: #71126
doc_auto_cfg
The tracking issue for this feature is: #43781
doc_cfg
The tracking issue for this feature is: #43781
The doc_cfg
feature allows an API be documented as only available in some specific platforms.
This attribute has two effects:
-
In the annotated item's documentation, there will be a message saying "This is supported on (platform) only".
-
The item's doc-tests will only run on the specific platform.
In addition to allowing the use of the #[doc(cfg)]
attribute, this feature enables the use of a
special conditional compilation flag, #[cfg(doc)]
, set whenever building documentation on your
crate.
This feature was introduced as part of PR #43348 to allow the platform-specific parts of the standard library be documented.
#![allow(unused)] #![feature(doc_cfg)] fn main() { #[cfg(any(windows, doc))] #[doc(cfg(windows))] /// The application's icon in the notification area (a.k.a. system tray). /// /// # Examples /// /// ```no_run /// extern crate my_awesome_ui_library; /// use my_awesome_ui_library::current_app; /// use my_awesome_ui_library::windows::notification; /// /// let icon = current_app().get::<notification::Icon>(); /// icon.show(); /// icon.show_message("Hello"); /// ``` pub struct Icon { // ... } }
doc_cfg_hide
The tracking issue for this feature is: #43781
doc_keyword
The tracking issue for this feature is: #51315
doc_masked
The tracking issue for this feature is: #44027
The doc_masked
feature allows a crate to exclude types from a given crate from appearing in lists
of trait implementations. The specifics of the feature are as follows:
-
When rustdoc encounters an
extern crate
statement annotated with a#[doc(masked)]
attribute, it marks the crate as being masked. -
When listing traits a given type implements, rustdoc ensures that traits from masked crates are not emitted into the documentation.
-
When listing types that implement a given trait, rustdoc ensures that types from masked crates are not emitted into the documentation.
This feature was introduced in PR #44026 to ensure that compiler-internal and implementation-specific types and traits were not included in the standard library's documentation. Such types would introduce broken links into the documentation.
doc_notable_trait
The tracking issue for this feature is: #45040
The doc_notable_trait
feature allows the use of the #[doc(notable_trait)]
attribute, which will display the trait in a "Notable traits" dialog for
functions returning types that implement the trait. For example, this attribute
is applied to the Iterator
, Future
, io::Read
, and io::Write
traits in
the standard library.
You can do this on your own traits like so:
#![feature(doc_notable_trait)]
#[doc(notable_trait)]
pub trait MyTrait {}
pub struct MyStruct;
impl MyTrait for MyStruct {}
/// The docs for this function will have a button that displays a dialog about
/// `MyStruct` implementing `MyTrait`.
pub fn my_fn() -> MyStruct { MyStruct }
This feature was originally implemented in PR #45039.
See also its documentation in the rustdoc book.
doc_primitive
The tracking issue for this feature is: #88070
dropck_eyepatch
The tracking issue for this feature is: #34761
ermsb_target_feature
The tracking issue for this feature is: #44839
exclusive_range_pattern
The tracking issue for this feature is: #37854.
The exclusive_range_pattern
feature allows non-inclusive range
patterns (0..10
) to be used in appropriate pattern matching
contexts. It also can be combined with #![feature(half_open_range_patterns]
to be able to use RangeTo patterns (..10
).
It also enabled RangeFrom patterns but that has since been stabilized.
#![allow(unused)] #![feature(exclusive_range_pattern)] fn main() { let x = 5; match x { 0..10 => println!("single digit"), 10 => println!("ten isn't part of the above range"), _ => println!("nor is everything else.") } }
exhaustive_patterns
The tracking issue for this feature is: #51085
explicit_generic_args_with_impl_trait
The tracking issue for this feature is: #83701
The explicit_generic_args_with_impl_trait
feature gate lets you specify generic arguments even
when impl Trait
is used in argument position.
A simple example is:
#![feature(explicit_generic_args_with_impl_trait)] fn foo<T: ?Sized>(_f: impl AsRef<T>) {} fn main() { foo::<str>("".to_string()); }
This is currently rejected:
error[E0632]: cannot provide explicit generic arguments when `impl Trait` is used in argument position
--> src/main.rs:6:11
|
6 | foo::<str>("".to_string());
| ^^^ explicit generic argument not allowed
However it would compile if explicit_generic_args_with_impl_trait
is enabled.
Note that the synthetic type parameters from impl Trait
are still implicit and you
cannot explicitly specify these:
#![feature(explicit_generic_args_with_impl_trait)] fn foo<T: ?Sized>(_f: impl AsRef<T>) {} fn bar<T: ?Sized, F: AsRef<T>>(_f: F) {} fn main() { bar::<str, _>("".to_string()); // Okay bar::<str, String>("".to_string()); // Okay foo::<str>("".to_string()); // Okay foo::<str, String>("".to_string()); // Error, you cannot specify `impl Trait` explicitly }
extern_types
The tracking issue for this feature is: #43467
f16c_target_feature
The tracking issue for this feature is: #44839
ffi_const
The tracking issue for this feature is: #58328
The #[ffi_const]
attribute applies clang's const
attribute to foreign
functions declarations.
That is, #[ffi_const]
functions shall have no effects except for its return
value, which can only depend on the values of the function parameters, and is
not affected by changes to the observable state of the program.
Applying the #[ffi_const]
attribute to a function that violates these
requirements is undefined behaviour.
This attribute enables Rust to perform common optimizations, like sub-expression
elimination, and it can avoid emitting some calls in repeated invocations of the
function with the same argument values regardless of other operations being
performed in between these functions calls (as opposed to #[ffi_pure]
functions).
Pitfalls
A #[ffi_const]
function can only read global memory that would not affect
its return value for the whole execution of the program (e.g. immutable global
memory). #[ffi_const]
functions are referentially-transparent and therefore
more strict than #[ffi_pure]
functions.
A common pitfall involves applying the #[ffi_const]
attribute to a
function that reads memory through pointer arguments which do not necessarily
point to immutable global memory.
A #[ffi_const]
function that returns unit has no effect on the abstract
machine's state, and a #[ffi_const]
function cannot be #[ffi_pure]
.
A #[ffi_const]
function must not diverge, neither via a side effect (e.g. a
call to abort
) nor by infinite loops.
When translating C headers to Rust FFI, it is worth verifying for which targets
the const
attribute is enabled in those headers, and using the appropriate
cfg
macros in the Rust side to match those definitions. While the semantics of
const
are implemented identically by many C and C++ compilers, e.g., clang,
GCC, ARM C/C++ compiler, IBM ILE C/C++, etc. they are not necessarily
implemented in this way on all of them. It is therefore also worth verifying
that the semantics of the C toolchain used to compile the binary being linked
against are compatible with those of the #[ffi_const]
.
ffi_pure
The tracking issue for this feature is: #58329
The #[ffi_pure]
attribute applies clang's pure
attribute to foreign
functions declarations.
That is, #[ffi_pure]
functions shall have no effects except for its return
value, which shall not change across two consecutive function calls with
the same parameters.
Applying the #[ffi_pure]
attribute to a function that violates these
requirements is undefined behavior.
This attribute enables Rust to perform common optimizations, like sub-expression
elimination and loop optimizations. Some common examples of pure functions are
strlen
or memcmp
.
These optimizations are only applicable when the compiler can prove that no
program state observable by the #[ffi_pure]
function has changed between calls
of the function, which could alter the result. See also the #[ffi_const]
attribute, which provides stronger guarantees regarding the allowable behavior
of a function, enabling further optimization.
Pitfalls
A #[ffi_pure]
function can read global memory through the function
parameters (e.g. pointers), globals, etc. #[ffi_pure]
functions are not
referentially-transparent, and are therefore more relaxed than #[ffi_const]
functions.
However, accessing global memory through volatile or atomic reads can violate the requirement that two consecutive function calls shall return the same value.
A pure
function that returns unit has no effect on the abstract machine's
state.
A #[ffi_pure]
function must not diverge, neither via a side effect (e.g. a
call to abort
) nor by infinite loops.
When translating C headers to Rust FFI, it is worth verifying for which targets
the pure
attribute is enabled in those headers, and using the appropriate
cfg
macros in the Rust side to match those definitions. While the semantics of
pure
are implemented identically by many C and C++ compilers, e.g., clang,
GCC, ARM C/C++ compiler, IBM ILE C/C++, etc. they are not necessarily
implemented in this way on all of them. It is therefore also worth verifying
that the semantics of the C toolchain used to compile the binary being linked
against are compatible with those of the #[ffi_pure]
.
ffi_returns_twice
The tracking issue for this feature is: #58314
fn_align
The tracking issue for this feature is: #82232
format_args_capture
The tracking issue for this feature is: #67984
fundamental
The tracking issue for this feature is: #29635
generators
The tracking issue for this feature is: #43122
The generators
feature gate in Rust allows you to define generator or
coroutine literals. A generator is a "resumable function" that syntactically
resembles a closure but compiles to much different semantics in the compiler
itself. The primary feature of a generator is that it can be suspended during
execution to be resumed at a later date. Generators use the yield
keyword to
"return", and then the caller can resume
a generator to resume execution just
after the yield
keyword.
Generators are an extra-unstable feature in the compiler right now. Added in RFC 2033 they're mostly intended right now as a information/constraint gathering phase. The intent is that experimentation can happen on the nightly compiler before actual stabilization. A further RFC will be required to stabilize generators/coroutines and will likely contain at least a few small tweaks to the overall design.
A syntactical example of a generator is:
#![feature(generators, generator_trait)] use std::ops::{Generator, GeneratorState}; use std::pin::Pin; fn main() { let mut generator = || { yield 1; return "foo" }; match Pin::new(&mut generator).resume(()) { GeneratorState::Yielded(1) => {} _ => panic!("unexpected value from resume"), } match Pin::new(&mut generator).resume(()) { GeneratorState::Complete("foo") => {} _ => panic!("unexpected value from resume"), } }
Generators are closure-like literals which can contain a yield
statement. The
yield
statement takes an optional expression of a value to yield out of the
generator. All generator literals implement the Generator
trait in the
std::ops
module. The Generator
trait has one main method, resume
, which
resumes execution of the generator at the previous suspension point.
An example of the control flow of generators is that the following example prints all numbers in order:
#![feature(generators, generator_trait)] use std::ops::Generator; use std::pin::Pin; fn main() { let mut generator = || { println!("2"); yield; println!("4"); }; println!("1"); Pin::new(&mut generator).resume(()); println!("3"); Pin::new(&mut generator).resume(()); println!("5"); }
At this time the main intended use case of generators is an implementation primitive for async/await syntax, but generators will likely be extended to ergonomic implementations of iterators and other primitives in the future. Feedback on the design and usage is always appreciated!
The Generator
trait
The Generator
trait in std::ops
currently looks like:
#![allow(unused)] fn main() { #![feature(arbitrary_self_types, generator_trait)] use std::ops::GeneratorState; use std::pin::Pin; pub trait Generator<R = ()> { type Yield; type Return; fn resume(self: Pin<&mut Self>, resume: R) -> GeneratorState<Self::Yield, Self::Return>; } }
The Generator::Yield
type is the type of values that can be yielded with the
yield
statement. The Generator::Return
type is the returned type of the
generator. This is typically the last expression in a generator's definition or
any value passed to return
in a generator. The resume
function is the entry
point for executing the Generator
itself.
The return value of resume
, GeneratorState
, looks like:
#![allow(unused)] fn main() { pub enum GeneratorState<Y, R> { Yielded(Y), Complete(R), } }
The Yielded
variant indicates that the generator can later be resumed. This
corresponds to a yield
point in a generator. The Complete
variant indicates
that the generator is complete and cannot be resumed again. Calling resume
after a generator has returned Complete
will likely result in a panic of the
program.
Closure-like semantics
The closure-like syntax for generators alludes to the fact that they also have closure-like semantics. Namely:
-
When created, a generator executes no code. A closure literal does not actually execute any of the closure's code on construction, and similarly a generator literal does not execute any code inside the generator when constructed.
-
Generators can capture outer variables by reference or by move, and this can be tweaked with the
move
keyword at the beginning of the closure. Like closures all generators will have an implicit environment which is inferred by the compiler. Outer variables can be moved into a generator for use as the generator progresses. -
Generator literals produce a value with a unique type which implements the
std::ops::Generator
trait. This allows actual execution of the generator through theGenerator::resume
method as well as also naming it in return types and such. -
Traits like
Send
andSync
are automatically implemented for aGenerator
depending on the captured variables of the environment. Unlike closures, generators also depend on variables live across suspension points. This means that although the ambient environment may beSend
orSync
, the generator itself may not be due to internal variables live acrossyield
points being not-Send
or not-Sync
. Note that generators do not implement traits likeCopy
orClone
automatically. -
Whenever a generator is dropped it will drop all captured environment variables.
Generators as state machines
In the compiler, generators are currently compiled as state machines. Each
yield
expression will correspond to a different state that stores all live
variables over that suspension point. Resumption of a generator will dispatch on
the current state and then execute internally until a yield
is reached, at
which point all state is saved off in the generator and a value is returned.
Let's take a look at an example to see what's going on here:
#![feature(generators, generator_trait)] use std::ops::Generator; use std::pin::Pin; fn main() { let ret = "foo"; let mut generator = move || { yield 1; return ret }; Pin::new(&mut generator).resume(()); Pin::new(&mut generator).resume(()); }
This generator literal will compile down to something similar to:
#![feature(arbitrary_self_types, generators, generator_trait)] use std::ops::{Generator, GeneratorState}; use std::pin::Pin; fn main() { let ret = "foo"; let mut generator = { enum __Generator { Start(&'static str), Yield1(&'static str), Done, } impl Generator for __Generator { type Yield = i32; type Return = &'static str; fn resume(mut self: Pin<&mut Self>, resume: ()) -> GeneratorState<i32, &'static str> { use std::mem; match mem::replace(&mut *self, __Generator::Done) { __Generator::Start(s) => { *self = __Generator::Yield1(s); GeneratorState::Yielded(1) } __Generator::Yield1(s) => { *self = __Generator::Done; GeneratorState::Complete(s) } __Generator::Done => { panic!("generator resumed after completion") } } } } __Generator::Start(ret) }; Pin::new(&mut generator).resume(()); Pin::new(&mut generator).resume(()); }
Notably here we can see that the compiler is generating a fresh type,
__Generator
in this case. This type has a number of states (represented here
as an enum
) corresponding to each of the conceptual states of the generator.
At the beginning we're closing over our outer variable foo
and then that
variable is also live over the yield
point, so it's stored in both states.
When the generator starts it'll immediately yield 1, but it saves off its state
just before it does so indicating that it has reached the yield point. Upon
resuming again we'll execute the return ret
which returns the Complete
state.
Here we can also note that the Done
state, if resumed, panics immediately as
it's invalid to resume a completed generator. It's also worth noting that this
is just a rough desugaring, not a normative specification for what the compiler
does.
generic_arg_infer
The tracking issue for this feature is: #85077
generic_associated_types
The tracking issue for this feature is: #44265
generic_const_exprs
The tracking issue for this feature is: #76560
half_open_range_patterns
The tracking issue for this feature is: #67264
It is part of the #![exclusive_range_pattern]
feature,
tracked at #37854.
The half_open_range_patterns
feature allows RangeTo patterns
(..10
) to be used in appropriate pattern matching contexts.
This requires also enabling the exclusive_range_pattern
feature.
It also enabled RangeFrom patterns but that has since been stabilized.
#![allow(unused)] #![feature(half_open_range_patterns)] #![feature(exclusive_range_pattern)] fn main() { let x = 5; match x { ..0 => println!("negative!"), // "RangeTo" pattern. Unstable. 0 => println!("zero!"), 1.. => println!("positive!"), // "RangeFrom" pattern. Stable. } }
hexagon_target_feature
The tracking issue for this feature is: #44839
if_let_guard
The tracking issue for this feature is: #51114
imported_main
The tracking issue for this feature is: #28937
in_band_lifetimes
The tracking issue for this feature is: #44524
infer_static_outlives_requirements
The tracking issue for this feature is: #54185
The infer_static_outlives_requirements
feature indicates that certain
'static
outlives requirements can be inferred by the compiler rather than
stating them explicitly.
Note: It is an accompanying feature to infer_outlives_requirements
,
which must be enabled to infer outlives requirements.
For example, currently generic struct definitions that contain references, require where-clauses of the form T: 'static. By using this feature the outlives predicates will be inferred, although they may still be written explicitly.
struct Foo<U> where U: 'static { // <-- currently required
bar: Bar<U>
}
struct Bar<T: 'static> {
x: T,
}
Examples:
#![feature(infer_outlives_requirements)]
#![feature(infer_static_outlives_requirements)]
#[rustc_outlives]
// Implicitly infer U: 'static
struct Foo<U> {
bar: Bar<U>
}
struct Bar<T: 'static> {
x: T,
}
inherent_associated_types
The tracking issue for this feature is: #8995
inline_const
The tracking issue for this feature is: #76001
This feature allows you to use inline constant expressions. For example, you can turn this code:
fn add_one(x: i32) -> i32 { x + 1 } const MY_COMPUTATION: i32 = 1 + 2 * 3 / 4; fn main() { let x = add_one(MY_COMPUTATION); }
into this code:
#![feature(inline_const)] fn add_one(x: i32) -> i32 { x + 1 } fn main() { let x = add_one(const { 1 + 2 * 3 / 4 }); }
You can also use inline constant expressions in patterns:
#![allow(unused)] #![feature(inline_const)] fn main() { const fn one() -> i32 { 1 } let some_int = 3; match some_int { const { 1 + 2 } => println!("Matched 1 + 2"), const { one() } => println!("Matched const fn returning 1"), _ => println!("Didn't match anything :("), } }
intra-doc-pointers
The tracking issue for this feature is: #80896
Rustdoc does not currently allow disambiguating between *const
and *mut
, and
raw pointers in intra-doc links are unstable until it does.
#![allow(unused)] #![feature(intra_doc_pointers)] fn main() { //! [pointer::add] }
intrinsics
The tracking issue for this feature is: None.
Intrinsics are never intended to be stable directly, but intrinsics are often exported in some sort of stable manner. Prefer using the stable interfaces to the intrinsic directly when you can.
These are imported as if they were FFI functions, with the special
rust-intrinsic
ABI. For example, if one was in a freestanding
context, but wished to be able to transmute
between types, and
perform efficient pointer arithmetic, one would import those functions
via a declaration like
#![feature(intrinsics)] fn main() {} extern "rust-intrinsic" { fn transmute<T, U>(x: T) -> U; fn offset<T>(dst: *const T, offset: isize) -> *const T; }
As with any other FFI functions, these are always unsafe
to call.
isa_attribute
The tracking issue for this feature is: #74727
label_break_value
The tracking issue for this feature is: #48594
lang_items
The tracking issue for this feature is: None.
The rustc
compiler has certain pluggable operations, that is,
functionality that isn't hard-coded into the language, but is
implemented in libraries, with a special marker to tell the compiler
it exists. The marker is the attribute #[lang = "..."]
and there are
various different values of ...
, i.e. various different 'lang
items'.
For example, Box
pointers require two lang items, one for allocation
and one for deallocation. A freestanding program that uses the Box
sugar for dynamic allocations via malloc
and free
:
#![feature(lang_items, box_syntax, start, libc, core_intrinsics, rustc_private)]
#![no_std]
use core::intrinsics;
use core::panic::PanicInfo;
extern crate libc;
#[lang = "owned_box"]
pub struct Box<T>(*mut T);
#[lang = "exchange_malloc"]
unsafe fn allocate(size: usize, _align: usize) -> *mut u8 {
let p = libc::malloc(size as libc::size_t) as *mut u8;
// Check if `malloc` failed:
if p as usize == 0 {
intrinsics::abort();
}
p
}
#[lang = "box_free"]
unsafe fn box_free<T: ?Sized>(ptr: *mut T) {
libc::free(ptr as *mut libc::c_void)
}
#[start]
fn main(_argc: isize, _argv: *const *const u8) -> isize {
let _x = box 1;
0
}
#[lang = "eh_personality"] extern fn rust_eh_personality() {}
#[lang = "panic_impl"] extern fn rust_begin_panic(info: &PanicInfo) -> ! { unsafe { intrinsics::abort() } }
#[no_mangle] pub extern fn rust_eh_register_frames () {}
#[no_mangle] pub extern fn rust_eh_unregister_frames () {}
Note the use of abort
: the exchange_malloc
lang item is assumed to
return a valid pointer, and so needs to do the check internally.
Other features provided by lang items include:
- overloadable operators via traits: the traits corresponding to the
==
,<
, dereferencing (*
) and+
(etc.) operators are all marked with lang items; those specific four areeq
,ord
,deref
, andadd
respectively. - stack unwinding and general failure; the
eh_personality
,panic
andpanic_bounds_check
lang items. - the traits in
std::marker
used to indicate types of various kinds; lang itemssend
,sync
andcopy
. - the marker types and variance indicators found in
std::marker
; lang itemscovariant_type
,contravariant_lifetime
, etc.
Lang items are loaded lazily by the compiler; e.g. if one never uses
Box
then there is no need to define functions for exchange_malloc
and box_free
. rustc
will emit an error when an item is needed
but not found in the current crate or any that it depends on.
Most lang items are defined by libcore
, but if you're trying to build
an executable without the standard library, you'll run into the need
for lang items. The rest of this page focuses on this use-case, even though
lang items are a bit broader than that.
Using libc
In order to build a #[no_std]
executable we will need libc as a dependency.
We can specify this using our Cargo.toml
file:
[dependencies]
libc = { version = "0.2.14", default-features = false }
Note that the default features have been disabled. This is a critical step - the default features of libc include the standard library and so must be disabled.
Writing an executable without stdlib
Controlling the entry point is possible in two ways: the #[start]
attribute,
or overriding the default shim for the C main
function with your own.
The function marked #[start]
is passed the command line parameters
in the same format as C:
#![feature(lang_items, core_intrinsics, rustc_private)]
#![feature(start)]
#![no_std]
use core::intrinsics;
use core::panic::PanicInfo;
// Pull in the system libc library for what crt0.o likely requires.
extern crate libc;
// Entry point for this program.
#[start]
fn start(_argc: isize, _argv: *const *const u8) -> isize {
0
}
// These functions are used by the compiler, but not
// for a bare-bones hello world. These are normally
// provided by libstd.
#[lang = "eh_personality"]
#[no_mangle]
pub extern fn rust_eh_personality() {
}
#[lang = "panic_impl"]
#[no_mangle]
pub extern fn rust_begin_panic(info: &PanicInfo) -> ! {
unsafe { intrinsics::abort() }
}
To override the compiler-inserted main
shim, one has to disable it
with #![no_main]
and then create the appropriate symbol with the
correct ABI and the correct name, which requires overriding the
compiler's name mangling too:
#![feature(lang_items, core_intrinsics, rustc_private)]
#![feature(start)]
#![no_std]
#![no_main]
use core::intrinsics;
use core::panic::PanicInfo;
// Pull in the system libc library for what crt0.o likely requires.
extern crate libc;
// Entry point for this program.
#[no_mangle] // ensure that this symbol is called `main` in the output
pub extern fn main(_argc: i32, _argv: *const *const u8) -> i32 {
0
}
// These functions are used by the compiler, but not
// for a bare-bones hello world. These are normally
// provided by libstd.
#[lang = "eh_personality"]
#[no_mangle]
pub extern fn rust_eh_personality() {
}
#[lang = "panic_impl"]
#[no_mangle]
pub extern fn rust_begin_panic(info: &PanicInfo) -> ! {
unsafe { intrinsics::abort() }
}
In many cases, you may need to manually link to the compiler_builtins
crate
when building a no_std
binary. You may observe this via linker error messages
such as "undefined reference to `__rust_probestack'
".
More about the language items
The compiler currently makes a few assumptions about symbols which are available in the executable to call. Normally these functions are provided by the standard library, but without it you must define your own. These symbols are called "language items", and they each have an internal name, and then a signature that an implementation must conform to.
The first of these functions, rust_eh_personality
, is used by the failure
mechanisms of the compiler. This is often mapped to GCC's personality function
(see the libstd implementation for more information), but crates
which do not trigger a panic can be assured that this function is never
called. The language item's name is eh_personality
.
The second function, rust_begin_panic
, is also used by the failure mechanisms of the
compiler. When a panic happens, this controls the message that's displayed on
the screen. While the language item's name is panic_impl
, the symbol name is
rust_begin_panic
.
Finally, a eh_catch_typeinfo
static is needed for certain targets which
implement Rust panics on top of C++ exceptions.
List of all language items
This is a list of all language items in Rust along with where they are located in the source code.
- Primitives
i8
:libcore/num/mod.rs
i16
:libcore/num/mod.rs
i32
:libcore/num/mod.rs
i64
:libcore/num/mod.rs
i128
:libcore/num/mod.rs
isize
:libcore/num/mod.rs
u8
:libcore/num/mod.rs
u16
:libcore/num/mod.rs
u32
:libcore/num/mod.rs
u64
:libcore/num/mod.rs
u128
:libcore/num/mod.rs
usize
:libcore/num/mod.rs
f32
:libstd/f32.rs
f64
:libstd/f64.rs
char
:libcore/char.rs
slice
:liballoc/slice.rs
str
:liballoc/str.rs
const_ptr
:libcore/ptr.rs
mut_ptr
:libcore/ptr.rs
unsafe_cell
:libcore/cell.rs
- Runtime
start
:libstd/rt.rs
eh_personality
:libpanic_unwind/emcc.rs
(EMCC)eh_personality
:libpanic_unwind/gcc.rs
(GNU)eh_personality
:libpanic_unwind/seh.rs
(SEH)eh_catch_typeinfo
:libpanic_unwind/emcc.rs
(EMCC)panic
:libcore/panicking.rs
panic_bounds_check
:libcore/panicking.rs
panic_impl
:libcore/panicking.rs
panic_impl
:libstd/panicking.rs
- Allocations
owned_box
:liballoc/boxed.rs
exchange_malloc
:liballoc/heap.rs
box_free
:liballoc/heap.rs
- Operands
not
:libcore/ops/bit.rs
bitand
:libcore/ops/bit.rs
bitor
:libcore/ops/bit.rs
bitxor
:libcore/ops/bit.rs
shl
:libcore/ops/bit.rs
shr
:libcore/ops/bit.rs
bitand_assign
:libcore/ops/bit.rs
bitor_assign
:libcore/ops/bit.rs
bitxor_assign
:libcore/ops/bit.rs
shl_assign
:libcore/ops/bit.rs
shr_assign
:libcore/ops/bit.rs
deref
:libcore/ops/deref.rs
deref_mut
:libcore/ops/deref.rs
index
:libcore/ops/index.rs
index_mut
:libcore/ops/index.rs
add
:libcore/ops/arith.rs
sub
:libcore/ops/arith.rs
mul
:libcore/ops/arith.rs
div
:libcore/ops/arith.rs
rem
:libcore/ops/arith.rs
neg
:libcore/ops/arith.rs
add_assign
:libcore/ops/arith.rs
sub_assign
:libcore/ops/arith.rs
mul_assign
:libcore/ops/arith.rs
div_assign
:libcore/ops/arith.rs
rem_assign
:libcore/ops/arith.rs
eq
:libcore/cmp.rs
ord
:libcore/cmp.rs
- Functions
fn
:libcore/ops/function.rs
fn_mut
:libcore/ops/function.rs
fn_once
:libcore/ops/function.rs
generator_state
:libcore/ops/generator.rs
generator
:libcore/ops/generator.rs
- Other
coerce_unsized
:libcore/ops/unsize.rs
drop
:libcore/ops/drop.rs
drop_in_place
:libcore/ptr.rs
clone
:libcore/clone.rs
copy
:libcore/marker.rs
send
:libcore/marker.rs
sized
:libcore/marker.rs
unsize
:libcore/marker.rs
sync
:libcore/marker.rs
phantom_data
:libcore/marker.rs
discriminant_kind
:libcore/marker.rs
freeze
:libcore/marker.rs
debug_trait
:libcore/fmt/mod.rs
non_zero
:libcore/nonzero.rs
arc
:liballoc/sync.rs
rc
:liballoc/rc.rs
large_assignments
The tracking issue for this feature is: #83518
let_chains
The tracking issue for this feature is: #53667
let_else
The tracking issue for this feature is: #87335
link_cfg
This feature is internal to the Rust compiler and is not intended for general use.
link_llvm_intrinsics
The tracking issue for this feature is: #29602
linkage
The tracking issue for this feature is: #29603
lint_reasons
The tracking issue for this feature is: #54503
marker_trait_attr
The tracking issue for this feature is: #29864
Normally, Rust keeps you from adding trait implementations that could overlap with each other, as it would be ambiguous which to use. This feature, however, carves out an exception to that rule: a trait can opt-in to having overlapping implementations, at the cost that those implementations are not allowed to override anything (and thus the trait itself cannot have any associated items, as they're pointless when they'd need to do the same thing for every type anyway).
#![allow(unused)] #![feature(marker_trait_attr)] fn main() { #[marker] trait CheapToClone: Clone {} impl<T: Copy> CheapToClone for T {} // These could potentially overlap with the blanket implementation above, // so are only allowed because CheapToClone is a marker trait. impl<T: CheapToClone, U: CheapToClone> CheapToClone for (T, U) {} impl<T: CheapToClone> CheapToClone for std::ops::Range<T> {} fn cheap_clone<T: CheapToClone>(t: T) -> T { t.clone() } }
This is expected to replace the unstable overlapping_marker_traits
feature, which applied to all empty traits (without needing an opt-in).
min_specialization
The tracking issue for this feature is: #31844
mips_target_feature
The tracking issue for this feature is: #44839
more_qualified_paths
The more_qualified_paths
feature can be used in order to enable the
use of qualified paths in patterns.
Example
#![feature(more_qualified_paths)] fn main() { // destructure through a qualified path let <Foo as A>::Assoc { br } = StructStruct { br: 2 }; } struct StructStruct { br: i8, } struct Foo; trait A { type Assoc; } impl A for Foo { type Assoc = StructStruct; }
movbe_target_feature
The tracking issue for this feature is: #44839
must_not_suspend
The tracking issue for this feature is: #83310
naked_functions
The tracking issue for this feature is: #32408
native_link_modifiers
The tracking issue for this feature is: #81490
The native_link_modifiers
feature allows you to use the modifiers
syntax with the #[link(..)]
attribute.
Modifiers are specified as a comma-delimited string with each modifier prefixed with either a +
or -
to indicate that the modifier is enabled or disabled, respectively. The last boolean value specified for a given modifier wins.
native_link_modifiers_as_needed
The tracking issue for this feature is: #81490
The native_link_modifiers_as_needed
feature allows you to use the as-needed
modifier.
as-needed
is only compatible with the dynamic
and framework
linking kinds. Using any other kind will result in a compiler error.
+as-needed
means that the library will be actually linked only if it satisfies some undefined symbols at the point at which it is specified on the command line, making it similar to static libraries in this regard.
This modifier translates to --as-needed
for ld-like linkers, and to -dead_strip_dylibs
/ -needed_library
/ -needed_framework
for ld64.
The modifier does nothing for linkers that don't support it (e.g. link.exe
).
The default for this modifier is unclear, some targets currently specify it as +as-needed
, some do not. We may want to try making +as-needed
a default for all targets.
native_link_modifiers_bundle
The tracking issue for this feature is: #81490
The native_link_modifiers_bundle
feature allows you to use the bundle
modifier.
Only compatible with the static
linking kind. Using any other kind will result in a compiler error.
+bundle
means objects from the static library are bundled into the produced crate (a rlib, for example) and are used from this crate later during linking of the final binary.
-bundle
means the static library is included into the produced rlib "by name" and object files from it are included only during linking of the final binary, the file search by that name is also performed during final linking.
This modifier is supposed to supersede the static-nobundle
linking kind defined by RFC 1717.
The default for this modifier is currently +bundle
, but it could be changed later on some future edition boundary.
native_link_modifiers_verbatim
The tracking issue for this feature is: #81490
The native_link_modifiers_verbatim
feature allows you to use the verbatim
modifier.
+verbatim
means that rustc itself won't add any target-specified library prefixes or suffixes (like lib
or .a
) to the library name, and will try its best to ask for the same thing from the linker.
For ld
-like linkers rustc will use the -l:filename
syntax (note the colon) when passing the library, so the linker won't add any prefixes or suffixes as well.
See -l namespec
in ld documentation for more details.
For linkers not supporting any verbatim modifiers (e.g. link.exe
or ld64
) the library name will be passed as is.
The default for this modifier is -verbatim
.
This RFC changes the behavior of raw-dylib
linking kind specified by RFC 2627. The .dll
suffix (or other target-specified suffixes for other targets) is now added automatically.
If your DLL doesn't have the .dll
suffix, it can be specified with +verbatim
.
native_link_modifiers_whole_archive
The tracking issue for this feature is: #81490
The native_link_modifiers_whole_archive
feature allows you to use the whole-archive
modifier.
Only compatible with the static
linking kind. Using any other kind will result in a compiler error.
+whole-archive
means that the static library is linked as a whole archive without throwing any object files away.
This modifier translates to --whole-archive
for ld
-like linkers, to /WHOLEARCHIVE
for link.exe
, and to -force_load
for ld64
.
The modifier does nothing for linkers that don't support it.
The default for this modifier is -whole-archive
.
needs_panic_runtime
The tracking issue for this feature is: #32837
negative_impls
The tracking issue for this feature is #68318.
With the feature gate negative_impls
, you can write negative impls as well as positive ones:
#![allow(unused)] #![feature(negative_impls)] fn main() { trait DerefMut { } impl<T: ?Sized> !DerefMut for &T { } }
Negative impls indicate a semver guarantee that the given trait will not be implemented for the given types. Negative impls play an additional purpose for auto traits, described below.
Negative impls have the following characteristics:
- They do not have any items.
- They must obey the orphan rules as if they were a positive impl.
- They cannot "overlap" with any positive impls.
Semver interaction
It is a breaking change to remove a negative impl. Negative impls are a commitment not to implement the given trait for the named types.
Orphan and overlap rules
Negative impls must obey the same orphan rules as a positive impl. This implies you cannot add a negative impl for types defined in upstream crates and so forth.
Similarly, negative impls cannot overlap with positive impls, again using the same "overlap" check that we ordinarily use to determine if two impls overlap. (Note that positive impls typically cannot overlap with one another either, except as permitted by specialization.)
Interaction with auto traits
Declaring a negative impl impl !SomeAutoTrait for SomeType
for an
auto-trait serves two purposes:
- as with any trait, it declares that
SomeType
will never implementSomeAutoTrait
; - it disables the automatic
SomeType: SomeAutoTrait
impl that would otherwise have been generated.
Note that, at present, there is no way to indicate that a given type
does not implement an auto trait but that it may do so in the
future. For ordinary types, this is done by simply not declaring any
impl at all, but that is not an option for auto traits. A workaround
is that one could embed a marker type as one of the fields, where the
marker type is !AutoTrait
.
Immediate uses
Negative impls are used to declare that &T: !DerefMut
and &mut T: !Clone
, as required to fix the soundness of Pin
described in #66544.
This serves two purposes:
- For proving the correctness of unsafe code, we can use that impl as evidence that no
DerefMut
orClone
impl exists. - It prevents downstream crates from creating such impls.
never_type
The tracking issue for this feature is: #35121
never_type_fallback
The tracking issue for this feature is: #65992
nll
The tracking issue for this feature is: #43234
no_core
The tracking issue for this feature is: #29639
no_coverage
The tracking issue for this feature is: #84605
The no_coverage
attribute can be used to selectively disable coverage
instrumentation in an annotated function. This might be useful to:
- Avoid instrumentation overhead in a performance critical function
- Avoid generating coverage for a function that is not meant to be executed, but still target 100% coverage for the rest of the program.
Example
#![allow(unused)] #![feature(no_coverage)] fn main() { // `foo()` will get coverage instrumentation (by default) fn foo() { // ... } #[no_coverage] fn bar() { // ... } }
no_niche
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
no_sanitize
The tracking issue for this feature is: #39699
The no_sanitize
attribute can be used to selectively disable sanitizer
instrumentation in an annotated function. This might be useful to: avoid
instrumentation overhead in a performance critical function, or avoid
instrumenting code that contains constructs unsupported by given sanitizer.
The precise effect of this annotation depends on particular sanitizer in use.
For example, with no_sanitize(thread)
, the thread sanitizer will no longer
instrument non-atomic store / load operations, but it will instrument atomic
operations to avoid reporting false positives and provide meaning full stack
traces.
Examples
#![allow(unused)] #![feature(no_sanitize)] fn main() { #[no_sanitize(address)] fn foo() { // ... } }
non_exhaustive_omitted_patterns_lint
The tracking issue for this feature is: #89554
object_safe_for_dispatch
The tracking issue for this feature is: #43561
omit_gdb_pretty_printer_section
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
optimize_attribute
The tracking issue for this feature is: #54882
panic_runtime
The tracking issue for this feature is: #32837
platform_intrinsics
The tracking issue for this feature is: #27731
plugin
The tracking issue for this feature is: #29597
This feature is part of "compiler plugins." It will often be used with the
rustc_private
feature.
rustc
can load compiler plugins, which are user-provided libraries that
extend the compiler's behavior with new lint checks, etc.
A plugin is a dynamic library crate with a designated registrar function that
registers extensions with rustc
. Other crates can load these extensions using
the crate attribute #![plugin(...)]
. See the
rustc_driver::plugin
documentation for more about the
mechanics of defining and loading a plugin.
In the vast majority of cases, a plugin should only be used through
#![plugin]
and not through an extern crate
item. Linking a plugin would
pull in all of librustc_ast and librustc as dependencies of your crate. This is
generally unwanted unless you are building another plugin.
The usual practice is to put compiler plugins in their own crate, separate from
any macro_rules!
macros or ordinary Rust code meant to be used by consumers
of a library.
Lint plugins
Plugins can extend Rust's lint
infrastructure with
additional checks for code style, safety, etc. Now let's write a plugin
lint-plugin-test.rs
that warns about any item named lintme
.
#![feature(box_syntax, rustc_private)]
extern crate rustc_ast;
// Load rustc as a plugin to get macros
extern crate rustc_driver;
#[macro_use]
extern crate rustc_lint;
#[macro_use]
extern crate rustc_session;
use rustc_driver::plugin::Registry;
use rustc_lint::{EarlyContext, EarlyLintPass, LintArray, LintContext, LintPass};
use rustc_ast::ast;
declare_lint!(TEST_LINT, Warn, "Warn about items named 'lintme'");
declare_lint_pass!(Pass => [TEST_LINT]);
impl EarlyLintPass for Pass {
fn check_item(&mut self, cx: &EarlyContext, it: &ast::Item) {
if it.ident.name.as_str() == "lintme" {
cx.lint(TEST_LINT, |lint| {
lint.build("item is named 'lintme'").set_span(it.span).emit()
});
}
}
}
#[no_mangle]
fn __rustc_plugin_registrar(reg: &mut Registry) {
reg.lint_store.register_lints(&[&TEST_LINT]);
reg.lint_store.register_early_pass(|| box Pass);
}
Then code like
#![feature(plugin)]
#![plugin(lint_plugin_test)]
fn lintme() { }
will produce a compiler warning:
foo.rs:4:1: 4:16 warning: item is named 'lintme', #[warn(test_lint)] on by default
foo.rs:4 fn lintme() { }
^~~~~~~~~~~~~~~
The components of a lint plugin are:
-
one or more
declare_lint!
invocations, which define staticLint
structs; -
a struct holding any state needed by the lint pass (here, none);
-
a
LintPass
implementation defining how to check each syntax element. A singleLintPass
may callspan_lint
for several differentLint
s, but should register them all through theget_lints
method.
Lint passes are syntax traversals, but they run at a late stage of compilation
where type information is available. rustc
's built-in
lints
mostly use the same infrastructure as lint plugins, and provide examples of how
to access type information.
Lints defined by plugins are controlled by the usual attributes and compiler
flags, e.g.
#[allow(test_lint)]
or -A test-lint
. These identifiers are derived from the
first argument to declare_lint!
, with appropriate case and punctuation
conversion.
You can run rustc -W help foo.rs
to see a list of lints known to rustc
,
including those provided by plugins loaded by foo.rs
.
powerpc_target_feature
The tracking issue for this feature is: #44839
precise_pointer_size_matching
The tracking issue for this feature is: #56354
prelude_import
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
proc_macro_hygiene
The tracking issue for this feature is: #54727
profiler_runtime
The tracking issue for this feature is: #42524.
raw_dylib
The tracking issue for this feature is: #58713
The raw_dylib
feature allows you to link against the implementations of functions in an extern
block without, on Windows, linking against an import library.
#![feature(raw_dylib)]
#[link(name="library", kind="raw-dylib")]
extern {
fn extern_function(x: i32);
}
fn main() {
unsafe {
extern_function(14);
}
}
Limitations
Currently, this feature is only supported on -windows-msvc
targets. Non-Windows platforms don't have import
libraries, and an incompatibility between LLVM and the BFD linker means that it is not currently supported on
-windows-gnu
targets.
On the i686-pc-windows-msvc
target, this feature supports only the cdecl
, stdcall
, system
, and fastcall
calling conventions.
raw_ref_op
The tracking issue for this feature is: #64490
register_attr
The tracking issue for this feature is: #66080
register_tool
The tracking issue for this feature is: #66079
relaxed_struct_unsize
The tracking issue for this feature is: #81793
repr_simd
The tracking issue for this feature is: #27731
repr128
The tracking issue for this feature is: #56071
The repr128
feature adds support for #[repr(u128)]
on enum
s.
#![allow(unused)] #![feature(repr128)] fn main() { #[repr(u128)] enum Foo { Bar(u64), } }
riscv_target_feature
The tracking issue for this feature is: #44839
rtm_target_feature
The tracking issue for this feature is: #44839
rustc_allow_const_fn_unstable
The tracking issue for this feature is: #69399
rustc_attrs
This feature has no tracking issue, and is therefore internal to the compiler, not being intended for general use.
Note: rustc_attrs
enables many rustc-internal attributes and this page
only discuss a few of them.
The rustc_attrs
feature allows debugging rustc type layouts by using
#[rustc_layout(...)]
to debug layout at compile time (it even works
with cargo check
) as an alternative to rustc -Z print-type-sizes
that is way more verbose.
Options provided by #[rustc_layout(...)]
are debug
, size
, align
,
abi
. Note that it only works on sized types without generics.
Examples
#![allow(unused)] #![feature(rustc_attrs)] fn main() { #[rustc_layout(abi, size)] pub enum X { Y(u8, u8, u8), Z(isize), } }
When that is compiled, the compiler will error with something like
error: abi: Aggregate { sized: true }
--> src/lib.rs:4:1
|
4 | / pub enum T {
5 | | Y(u8, u8, u8),
6 | | Z(isize),
7 | | }
| |_^
error: size: Size { raw: 16 }
--> src/lib.rs:4:1
|
4 | / pub enum T {
5 | | Y(u8, u8, u8),
6 | | Z(isize),
7 | | }
| |_^
error: aborting due to 2 previous errors
rustc_private
The tracking issue for this feature is: #27812
simd_ffi
The tracking issue for this feature is: #27731
specialization
The tracking issue for this feature is: #31844
sse4a_target_feature
The tracking issue for this feature is: #44839
staged_api
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
start
The tracking issue for this feature is: #29633
static_nobundle
The tracking issue for this feature is: #37403
stmt_expr_attributes
The tracking issue for this feature is: #15701
structural_match
The tracking issue for this feature is: #31434
target_feature_11
The tracking issue for this feature is: #69098
tbm_target_feature
The tracking issue for this feature is: #44839
test_2018_feature
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
thread_local
The tracking issue for this feature is: #29594
trait_alias
The tracking issue for this feature is: #41517
The trait_alias
feature adds support for trait aliases. These allow aliases
to be created for one or more traits (currently just a single regular trait plus
any number of auto-traits), and used wherever traits would normally be used as
either bounds or trait objects.
#![feature(trait_alias)] trait Foo = std::fmt::Debug + Send; trait Bar = Foo + Sync; // Use trait alias as bound on type parameter. fn foo<T: Foo>(v: &T) { println!("{:?}", v); } pub fn main() { foo(&1); // Use trait alias for trait objects. let a: &Bar = &123; println!("{:?}", a); let b = Box::new(456) as Box<dyn Foo>; println!("{:?}", b); }
trait_upcasting
The tracking issue for this feature is: #65991
The trait_upcasting
feature adds support for trait upcasting coercion. This allows a
trait object of type dyn Bar
to be cast to a trait object of type dyn Foo
so long as Bar: Foo
.
#![allow(unused)] #![feature(trait_upcasting)] #![allow(incomplete_features)] fn main() { trait Foo {} trait Bar: Foo {} impl Foo for i32 {} impl<T: Foo + ?Sized> Bar for T {} let bar: &dyn Bar = &123; let foo: &dyn Foo = bar; }
transparent_unions
The tracking issue for this feature is #60405
The transparent_unions
feature allows you mark union
s as
#[repr(transparent)]
. A union
may be #[repr(transparent)]
in exactly the
same conditions in which a struct
may be #[repr(transparent)]
(generally,
this means the union
must have exactly one non-zero-sized field). Some
concrete illustrations follow.
#![allow(unused)] #![feature(transparent_unions)] fn main() { // This union has the same representation as `f32`. #[repr(transparent)] union SingleFieldUnion { field: f32, } // This union has the same representation as `usize`. #[repr(transparent)] union MultiFieldUnion { field: usize, nothing: (), } }
For consistency with transparent struct
s, union
s must have exactly one
non-zero-sized field. If all fields are zero-sized, the union
must not be
#[repr(transparent)]
:
#![allow(unused)] #![feature(transparent_unions)] fn main() { // This (non-transparent) union is already valid in stable Rust: pub union GoodUnion { pub nothing: (), } // Error: transparent union needs exactly one non-zero-sized field, but has 0 // #[repr(transparent)] // pub union BadUnion { // pub nothing: (), // } }
The one exception is if the union
is generic over T
and has a field of type
T
, it may be #[repr(transparent)]
even if T
is a zero-sized type:
#![allow(unused)] #![feature(transparent_unions)] fn main() { // This union has the same representation as `T`. #[repr(transparent)] pub union GenericUnion<T: Copy> { // Unions with non-`Copy` fields are unstable. pub field: T, pub nothing: (), } // This is okay even though `()` is a zero-sized type. pub const THIS_IS_OKAY: GenericUnion<()> = GenericUnion { field: () }; }
Like transarent struct
s, a transparent union
of type U
has the same
layout, size, and ABI as its single non-ZST field. If it is generic over a type
T
, and all its fields are ZSTs except for exactly one field of type T
, then
it has the same layout and ABI as T
(even if T
is a ZST when monomorphized).
Like transparent struct
s, transparent union
s are FFI-safe if and only if
their underlying representation type is also FFI-safe.
A union
may not be eligible for the same nonnull-style optimizations that a
struct
or enum
(with the same fields) are eligible for. Adding
#[repr(transparent)]
to union
does not change this. To give a more concrete
example, it is unspecified whether size_of::<T>()
is equal to
size_of::<Option<T>>()
, where T
is a union
(regardless of whether or not
it is transparent). The Rust compiler is free to perform this optimization if
possible, but is not required to, and different compiler versions may differ in
their application of these optimizations.
trivial_bounds
The tracking issue for this feature is: #48214
try_blocks
The tracking issue for this feature is: #31436
The try_blocks
feature adds support for try
blocks. A try
block creates a new scope one can use the ?
operator in.
#![allow(unused)] #![feature(try_blocks)] fn main() { use std::num::ParseIntError; let result: Result<i32, ParseIntError> = try { "1".parse::<i32>()? + "2".parse::<i32>()? + "3".parse::<i32>()? }; assert_eq!(result, Ok(6)); let result: Result<i32, ParseIntError> = try { "1".parse::<i32>()? + "foo".parse::<i32>()? + "3".parse::<i32>()? }; assert!(result.is_err()); }
type_alias_impl_trait
The tracking issue for this feature is: #63063
type_ascription
The tracking issue for this feature is: #23416
unboxed_closures
The tracking issue for this feature is #29625
See Also: fn_traits
The unboxed_closures
feature allows you to write functions using the "rust-call"
ABI,
required for implementing the Fn*
family of traits. "rust-call"
functions must have
exactly one (non self) argument, a tuple representing the argument list.
#![feature(unboxed_closures)] extern "rust-call" fn add_args(args: (u32, u32)) -> u32 { args.0 + args.1 } fn main() {}
unsized_fn_params
The tracking issue for this feature is: #48055
unsized_locals
The tracking issue for this feature is: #48055
This implements RFC1909. When turned on, you can have unsized arguments and locals:
#![allow(incomplete_features)] #![feature(unsized_locals, unsized_fn_params)] use std::any::Any; fn main() { let x: Box<dyn Any> = Box::new(42); let x: dyn Any = *x; // ^ unsized local variable // ^^ unsized temporary foo(x); } fn foo(_: dyn Any) {} // ^^^^^^ unsized argument
The RFC still forbids the following unsized expressions:
#![feature(unsized_locals)] use std::any::Any; struct MyStruct<T: ?Sized> { content: T, } struct MyTupleStruct<T: ?Sized>(T); fn answer() -> Box<dyn Any> { Box::new(42) } fn main() { // You CANNOT have unsized statics. static X: dyn Any = *answer(); // ERROR const Y: dyn Any = *answer(); // ERROR // You CANNOT have struct initialized unsized. MyStruct { content: *answer() }; // ERROR MyTupleStruct(*answer()); // ERROR (42, *answer()); // ERROR // You CANNOT have unsized return types. fn my_function() -> dyn Any { *answer() } // ERROR // You CAN have unsized local variables... let mut x: dyn Any = *answer(); // OK // ...but you CANNOT reassign to them. x = *answer(); // ERROR // You CANNOT even initialize them separately. let y: dyn Any; // OK y = *answer(); // ERROR // Not mentioned in the RFC, but by-move captured variables are also Sized. let x: dyn Any = *answer(); (move || { // ERROR let y = x; })(); // You CAN create a closure with unsized arguments, // but you CANNOT call it. // This is an implementation detail and may be changed in the future. let f = |x: dyn Any| {}; f(*answer()); // ERROR }
By-value trait objects
With this feature, you can have by-value self
arguments without Self: Sized
bounds.
#![feature(unsized_fn_params)] trait Foo { fn foo(self) {} } impl<T: ?Sized> Foo for T {} fn main() { let slice: Box<[i32]> = Box::new([1, 2, 3]); <[i32] as Foo>::foo(*slice); }
And Foo
will also be object-safe.
#![feature(unsized_fn_params)] trait Foo { fn foo(self) {} } impl<T: ?Sized> Foo for T {} fn main () { let slice: Box<dyn Foo> = Box::new([1, 2, 3]); // doesn't compile yet <dyn Foo as Foo>::foo(*slice); }
One of the objectives of this feature is to allow Box<dyn FnOnce>
.
Variable length arrays
The RFC also describes an extension to the array literal syntax: [e; dyn n]
. In the syntax, n
isn't necessarily a constant expression. The array is dynamically allocated on the stack and has the type of [T]
, instead of [T; n]
.
#![feature(unsized_locals)]
fn mergesort<T: Ord>(a: &mut [T]) {
let mut tmp = [T; dyn a.len()];
// ...
}
fn main() {
let mut a = [3, 1, 5, 6];
mergesort(&mut a);
assert_eq!(a, [1, 3, 5, 6]);
}
VLAs are not implemented yet. The syntax isn't final, either. We may need an alternative syntax for Rust 2015 because, in Rust 2015, expressions like [e; dyn(1)]
would be ambiguous. One possible alternative proposed in the RFC is [e; n]
: if n
captures one or more local variables, then it is considered as [e; dyn n]
.
Advisory on stack usage
It's advised not to casually use the #![feature(unsized_locals)]
feature. Typical use-cases are:
- When you need a by-value trait objects.
- When you really need a fast allocation of small temporary arrays.
Another pitfall is repetitive allocation and temporaries. Currently the compiler simply extends the stack frame every time it encounters an unsized assignment. So for example, the code
#![feature(unsized_locals)] fn main() { let x: Box<[i32]> = Box::new([1, 2, 3, 4, 5]); let _x = {{{{{{{{{{*x}}}}}}}}}}; }
and the code
#![feature(unsized_locals)] fn main() { for _ in 0..10 { let x: Box<[i32]> = Box::new([1, 2, 3, 4, 5]); let _x = *x; } }
will unnecessarily extend the stack frame.
unsized_tuple_coercion
The tracking issue for this feature is: #42877
This is a part of RFC0401. According to the RFC, there should be an implementation like this:
impl<..., T, U: ?Sized> Unsized<(..., U)> for (..., T) where T: Unsized<U> {}
This implementation is currently gated behind #[feature(unsized_tuple_coercion)]
to avoid insta-stability. Therefore you can use it like this:
#![feature(unsized_tuple_coercion)] fn main() { let x : ([i32; 3], [i32; 3]) = ([1, 2, 3], [4, 5, 6]); let y : &([i32; 3], [i32]) = &x; assert_eq!(y.1[0], 4); }
untagged_unions
The tracking issue for this feature is: #55149
wasm_abi
The tracking issue for this feature is: #83788
wasm_target_feature
The tracking issue for this feature is: #44839
Library Features
alloc_error_hook
The tracking issue for this feature is: #51245
alloc_internals
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
alloc_layout_extra
The tracking issue for this feature is: #55724
allocator_api
The tracking issue for this feature is #32838
Sometimes you want the memory for one collection to use a different
allocator than the memory for another collection. In this case,
replacing the global allocator is not a workable option. Instead,
you need to pass in an instance of an AllocRef
to each collection
for which you want a custom allocator.
TBD
arc_new_cyclic
The tracking issue for this feature is: #75861
array_chunks
The tracking issue for this feature is: #74985
array_error_internals
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
array_from_fn
The tracking issue for this feature is: #89379
array_methods
The tracking issue for this feature is: #76118
array_windows
The tracking issue for this feature is: #75027
array_zip
The tracking issue for this feature is: #80094
as_array_of_cells
The tracking issue for this feature is: #88248
asm
The tracking issue for this feature is: #72016
For extremely low-level manipulations and performance reasons, one
might wish to control the CPU directly. Rust supports using inline
assembly to do this via the asm!
macro.
Guide-level explanation
Rust provides support for inline assembly via the asm!
macro.
It can be used to embed handwritten assembly in the assembly output generated by the compiler.
Generally this should not be necessary, but might be where the required performance or timing
cannot be otherwise achieved. Accessing low level hardware primitives, e.g. in kernel code, may also demand this functionality.
Note: the examples here are given in x86/x86-64 assembly, but other architectures are also supported.
Inline assembly is currently supported on the following architectures:
- x86 and x86-64
- ARM
- AArch64
- RISC-V
- NVPTX
- PowerPC
- Hexagon
- MIPS32r2 and MIPS64r2
- wasm32
- BPF
- SPIR-V
Basic usage
Let us start with the simplest possible example:
#![allow(unused)] #![feature(asm)] fn main() { unsafe { asm!("nop"); } }
This will insert a NOP (no operation) instruction into the assembly generated by the compiler.
Note that all asm!
invocations have to be inside an unsafe
block, as they could insert
arbitrary instructions and break various invariants. The instructions to be inserted are listed
in the first argument of the asm!
macro as a string literal.
Inputs and outputs
Now inserting an instruction that does nothing is rather boring. Let us do something that actually acts on data:
#![allow(unused)] #![feature(asm)] fn main() { let x: u64; unsafe { asm!("mov {}, 5", out(reg) x); } assert_eq!(x, 5); }
This will write the value 5
into the u64
variable x
.
You can see that the string literal we use to specify instructions is actually a template string.
It is governed by the same rules as Rust format strings.
The arguments that are inserted into the template however look a bit different then you may
be familiar with. First we need to specify if the variable is an input or an output of the
inline assembly. In this case it is an output. We declared this by writing out
.
We also need to specify in what kind of register the assembly expects the variable.
In this case we put it in an arbitrary general purpose register by specifying reg
.
The compiler will choose an appropriate register to insert into
the template and will read the variable from there after the inline assembly finishes executing.
Let us see another example that also uses an input:
#![allow(unused)] #![feature(asm)] fn main() { let i: u64 = 3; let o: u64; unsafe { asm!( "mov {0}, {1}", "add {0}, {number}", out(reg) o, in(reg) i, number = const 5, ); } assert_eq!(o, 8); }
This will add 5
to the input in variable i
and write the result to variable o
.
The particular way this assembly does this is first copying the value from i
to the output,
and then adding 5
to it.
The example shows a few things:
First, we can see that asm!
allows multiple template string arguments; each
one is treated as a separate line of assembly code, as if they were all joined
together with newlines between them. This makes it easy to format assembly
code.
Second, we can see that inputs are declared by writing in
instead of out
.
Third, one of our operands has a type we haven't seen yet, const
.
This tells the compiler to expand this argument to value directly inside the assembly template.
This is only possible for constants and literals.
Fourth, we can see that we can specify an argument number, or name as in any format string. For inline assembly templates this is particularly useful as arguments are often used more than once. For more complex inline assembly using this facility is generally recommended, as it improves readability, and allows reordering instructions without changing the argument order.
We can further refine the above example to avoid the mov
instruction:
#![allow(unused)] #![feature(asm)] fn main() { let mut x: u64 = 3; unsafe { asm!("add {0}, {number}", inout(reg) x, number = const 5); } assert_eq!(x, 8); }
We can see that inout
is used to specify an argument that is both input and output.
This is different from specifying an input and output separately in that it is guaranteed to assign both to the same register.
It is also possible to specify different variables for the input and output parts of an inout
operand:
#![allow(unused)] #![feature(asm)] fn main() { let x: u64 = 3; let y: u64; unsafe { asm!("add {0}, {number}", inout(reg) x => y, number = const 5); } assert_eq!(y, 8); }
Late output operands
The Rust compiler is conservative with its allocation of operands. It is assumed that an out
can be written at any time, and can therefore not share its location with any other argument.
However, to guarantee optimal performance it is important to use as few registers as possible,
so they won't have to be saved and reloaded around the inline assembly block.
To achieve this Rust provides a lateout
specifier. This can be used on any output that is
written only after all inputs have been consumed.
There is also a inlateout
variant of this specifier.
Here is an example where inlateout
cannot be used:
#![allow(unused)] #![feature(asm)] fn main() { let mut a: u64 = 4; let b: u64 = 4; let c: u64 = 4; unsafe { asm!( "add {0}, {1}", "add {0}, {2}", inout(reg) a, in(reg) b, in(reg) c, ); } assert_eq!(a, 12); }
Here the compiler is free to allocate the same register for inputs b
and c
since it knows they have the same value. However it must allocate a separate register for a
since it uses inout
and not inlateout
. If inlateout
was used, then a
and c
could be allocated to the same register, in which case the first instruction to overwrite the value of c
and cause the assembly code to produce the wrong result.
However the following example can use inlateout
since the output is only modified after all input registers have been read:
#![allow(unused)] #![feature(asm)] fn main() { let mut a: u64 = 4; let b: u64 = 4; unsafe { asm!("add {0}, {1}", inlateout(reg) a, in(reg) b); } assert_eq!(a, 8); }
As you can see, this assembly fragment will still work correctly if a
and b
are assigned to the same register.
Explicit register operands
Some instructions require that the operands be in a specific register.
Therefore, Rust inline assembly provides some more specific constraint specifiers.
While reg
is generally available on any architecture, explicit registers are highly architecture specific. E.g. for x86 the general purpose registers eax
, ebx
, ecx
, edx
, ebp
, esi
, and edi
among others can be addressed by their name.
#![allow(unused)] #![feature(asm)] fn main() { let cmd = 0xd1; unsafe { asm!("out 0x64, eax", in("eax") cmd); } }
In this example we call the out
instruction to output the content of the cmd
variable to port 0x64
. Since the out
instruction only accepts eax
(and its sub registers) as operand we had to use the eax
constraint specifier.
Note: unlike other operand types, explicit register operands cannot be used in the template string: you can't use
{}
and should write the register name directly instead. Also, they must appear at the end of the operand list after all other operand types.
Consider this example which uses the x86 mul
instruction:
#![allow(unused)] #![feature(asm)] fn main() { fn mul(a: u64, b: u64) -> u128 { let lo: u64; let hi: u64; unsafe { asm!( // The x86 mul instruction takes rax as an implicit input and writes // the 128-bit result of the multiplication to rax:rdx. "mul {}", in(reg) a, inlateout("rax") b => lo, lateout("rdx") hi ); } ((hi as u128) << 64) + lo as u128 } }
This uses the mul
instruction to multiply two 64-bit inputs with a 128-bit result.
The only explicit operand is a register, that we fill from the variable a
.
The second operand is implicit, and must be the rax
register, which we fill from the variable b
.
The lower 64 bits of the result are stored in rax
from which we fill the variable lo
.
The higher 64 bits are stored in rdx
from which we fill the variable hi
.
Clobbered registers
In many cases inline assembly will modify state that is not needed as an output. Usually this is either because we have to use a scratch register in the assembly or because instructions modify state that we don't need to further examine. This state is generally referred to as being "clobbered". We need to tell the compiler about this since it may need to save and restore this state around the inline assembly block.
#![allow(unused)] #![feature(asm)] fn main() { let ebx: u32; let ecx: u32; unsafe { asm!( "cpuid", // EAX 4 selects the "Deterministic Cache Parameters" CPUID leaf inout("eax") 4 => _, // ECX 0 selects the L0 cache information. inout("ecx") 0 => ecx, lateout("ebx") ebx, lateout("edx") _, ); } println!( "L1 Cache: {}", ((ebx >> 22) + 1) * (((ebx >> 12) & 0x3ff) + 1) * ((ebx & 0xfff) + 1) * (ecx + 1) ); }
In the example above we use the cpuid
instruction to get the L1 cache size.
This instruction writes to eax
, ebx
, ecx
, and edx
, but for the cache size we only care about the contents of ebx
and ecx
.
However we still need to tell the compiler that eax
and edx
have been modified so that it can save any values that were in these registers before the asm. This is done by declaring these as outputs but with _
instead of a variable name, which indicates that the output value is to be discarded.
This can also be used with a general register class (e.g. reg
) to obtain a scratch register for use inside the asm code:
#![allow(unused)] #![feature(asm)] fn main() { // Multiply x by 6 using shifts and adds let mut x: u64 = 4; unsafe { asm!( "mov {tmp}, {x}", "shl {tmp}, 1", "shl {x}, 2", "add {x}, {tmp}", x = inout(reg) x, tmp = out(reg) _, ); } assert_eq!(x, 4 * 6); }
Symbol operands and ABI clobbers
A special operand type, sym
, allows you to use the symbol name of a fn
or static
in inline assembly code.
This allows you to call a function or access a global variable without needing to keep its address in a register.
#![allow(unused)] #![feature(asm)] fn main() { extern "C" fn foo(arg: i32) -> i32 { println!("arg = {}", arg); arg * 2 } fn call_foo(arg: i32) -> i32 { unsafe { let result; asm!( "call {}", sym foo, // 1st argument in rdi in("rdi") arg, // Return value in rax out("rax") result, // Mark all registers which are not preserved by the "C" calling // convention as clobbered. clobber_abi("C"), ); result } } }
Note that the fn
or static
item does not need to be public or #[no_mangle]
: the compiler will automatically insert the appropriate mangled symbol name into the assembly code.
By default, asm!
assumes that any register not specified as an output will have its contents preserved by the assembly code. The clobber_abi
argument to asm!
tells the compiler to automatically insert the necessary clobber operands according to the given calling convention ABI: any register which is not fully preserved in that ABI will be treated as clobbered.
Register template modifiers
In some cases, fine control is needed over the way a register name is formatted when inserted into the template string. This is needed when an architecture's assembly language has several names for the same register, each typically being a "view" over a subset of the register (e.g. the low 32 bits of a 64-bit register).
By default the compiler will always choose the name that refers to the full register size (e.g. rax
on x86-64, eax
on x86, etc).
This default can be overriden by using modifiers on the template string operands, just like you would with format strings:
#![allow(unused)] #![feature(asm)] fn main() { let mut x: u16 = 0xab; unsafe { asm!("mov {0:h}, {0:l}", inout(reg_abcd) x); } assert_eq!(x, 0xabab); }
In this example, we use the reg_abcd
register class to restrict the register allocator to the 4 legacy x86 register (ax
, bx
, cx
, dx
) of which the first two bytes can be addressed independently.
Let us assume that the register allocator has chosen to allocate x
in the ax
register.
The h
modifier will emit the register name for the high byte of that register and the l
modifier will emit the register name for the low byte. The asm code will therefore be expanded as mov ah, al
which copies the low byte of the value into the high byte.
If you use a smaller data type (e.g. u16
) with an operand and forget the use template modifiers, the compiler will emit a warning and suggest the correct modifier to use.
Memory address operands
Sometimes assembly instructions require operands passed via memory addresses/memory locations.
You have to manually use the memory address syntax specified by the target architecture.
For example, on x86/x86_64 using intel assembly syntax, you should wrap inputs/outputs in []
to indicate they are memory operands:
#![allow(unused)] #![feature(asm, llvm_asm)] fn main() { fn load_fpu_control_word(control: u16) { unsafe { asm!("fldcw [{}]", in(reg) &control, options(nostack)); // Previously this would have been written with the deprecated `llvm_asm!` like this llvm_asm!("fldcw $0" :: "m" (control) :: "volatile"); } } }
Labels
Any reuse of a named label, local or otherwise, can result in a assembler or linker error or may cause other strange behavior. Reuse of a named label can happen in a variety of ways including:
- explicitly: using a label more than once in one
asm!
block, or multiple times across blocks - implicitly via inlining: the compiler is allowed to instantiate multiple copies of an
asm!
block, for example when the function containing it is inlined in multiple places. - implicitly via LTO: LTO can cause code from other crates to be placed in the same codegen unit, and so could bring in arbitrary labels
As a consequence, you should only use GNU assembler numeric local labels inside inline assembly code. Defining symbols in assembly code may lead to assembler and/or linker errors due to duplicate symbol definitions.
Moreover, on x86 when using the default intel syntax, due to an llvm bug, you shouldn't use labels exclusively made of 0
and 1
digits, e.g. 0
, 11
or 101010
, as they may end up being interpreted as binary values. Using options(att_syntax)
will avoid any ambiguity, but that affects the syntax of the entire asm!
block.
#![allow(unused)] #![feature(asm)] fn main() { let mut a = 0; unsafe { asm!( "mov {0}, 10", "2:", "sub {0}, 1", "cmp {0}, 3", "jle 2f", "jmp 2b", "2:", "add {0}, 2", out(reg) a ); } assert_eq!(a, 5); }
This will decrement the {0}
register value from 10 to 3, then add 2 and store it in a
.
This example shows a few things:
First that the same number can be used as a label multiple times in the same inline block.
Second, that when a numeric label is used as a reference (as an instruction operand, for example), the suffixes b (“backward”) or f (“forward”) should be added to the numeric label. It will then refer to the nearest label defined by this number in this direction.
Options
By default, an inline assembly block is treated the same way as an external FFI function call with a custom calling convention: it may read/write memory, have observable side effects, etc. However, in many cases it is desirable to give the compiler more information about what the assembly code is actually doing so that it can optimize better.
Let's take our previous example of an add
instruction:
#![allow(unused)] #![feature(asm)] fn main() { let mut a: u64 = 4; let b: u64 = 4; unsafe { asm!( "add {0}, {1}", inlateout(reg) a, in(reg) b, options(pure, nomem, nostack), ); } assert_eq!(a, 8); }
Options can be provided as an optional final argument to the asm!
macro. We specified three options here:
pure
means that the asm code has no observable side effects and that its output depends only on its inputs. This allows the compiler optimizer to call the inline asm fewer times or even eliminate it entirely.nomem
means that the asm code does not read or write to memory. By default the compiler will assume that inline assembly can read or write any memory address that is accessible to it (e.g. through a pointer passed as an operand, or a global).nostack
means that the asm code does not push any data onto the stack. This allows the compiler to use optimizations such as the stack red zone on x86-64 to avoid stack pointer adjustments.
These allow the compiler to better optimize code using asm!
, for example by eliminating pure asm!
blocks whose outputs are not needed.
See the reference for the full list of available options and their effects.
Reference-level explanation
Inline assembler is implemented as an unsafe macro asm!()
.
The first argument to this macro is a template string literal used to build the final assembly.
The following arguments specify input and output operands.
When required, options are specified as the final argument.
The following ABNF specifies the general syntax:
dir_spec := "in" / "out" / "lateout" / "inout" / "inlateout"
reg_spec := <register class> / "<explicit register>"
operand_expr := expr / "_" / expr "=>" expr / expr "=>" "_"
reg_operand := dir_spec "(" reg_spec ")" operand_expr
operand := reg_operand / "const" const_expr / "sym" path
clobber_abi := "clobber_abi(" <abi> ")"
option := "pure" / "nomem" / "readonly" / "preserves_flags" / "noreturn" / "nostack" / "att_syntax" / "raw"
options := "options(" option *["," option] [","] ")"
asm := "asm!(" format_string *("," format_string) *("," [ident "="] operand) ["," clobber_abi] *("," options) [","] ")"
Inline assembly is currently supported on the following architectures:
- x86 and x86-64
- ARM
- AArch64
- RISC-V
- NVPTX
- PowerPC
- Hexagon
- MIPS32r2 and MIPS64r2
- wasm32
- BPF
- SPIR-V
Support for more targets may be added in the future. The compiler will emit an error if asm!
is used on an unsupported target.
Template string arguments
The assembler template uses the same syntax as format strings (i.e. placeholders are specified by curly braces). The corresponding arguments are accessed in order, by index, or by name. However, implicit named arguments (introduced by RFC #2795) are not supported.
An asm!
invocation may have one or more template string arguments; an asm!
with multiple template string arguments is treated as if all the strings were concatenated with a \n
between them. The expected usage is for each template string argument to correspond to a line of assembly code. All template string arguments must appear before any other arguments.
As with format strings, named arguments must appear after positional arguments. Explicit register operands must appear at the end of the operand list, after named arguments if any.
Explicit register operands cannot be used by placeholders in the template string. All other named and positional operands must appear at least once in the template string, otherwise a compiler error is generated.
The exact assembly code syntax is target-specific and opaque to the compiler except for the way operands are substituted into the template string to form the code passed to the assembler.
The 5 targets specified in this RFC (x86, ARM, AArch64, RISC-V, Hexagon) all use the assembly code syntax of the GNU assembler (GAS). On x86, the .intel_syntax noprefix
mode of GAS is used by default. On ARM, the .syntax unified
mode is used. These targets impose an additional restriction on the assembly code: any assembler state (e.g. the current section which can be changed with .section
) must be restored to its original value at the end of the asm string. Assembly code that does not conform to the GAS syntax will result in assembler-specific behavior.
Operand type
Several types of operands are supported:
in(<reg>) <expr>
<reg>
can refer to a register class or an explicit register. The allocated register name is substituted into the asm template string.- The allocated register will contain the value of
<expr>
at the start of the asm code. - The allocated register must contain the same value at the end of the asm code (except if a
lateout
is allocated to the same register).
out(<reg>) <expr>
<reg>
can refer to a register class or an explicit register. The allocated register name is substituted into the asm template string.- The allocated register will contain an undefined value at the start of the asm code.
<expr>
must be a (possibly uninitialized) place expression, to which the contents of the allocated register is written to at the end of the asm code.- An underscore (
_
) may be specified instead of an expression, which will cause the contents of the register to be discarded at the end of the asm code (effectively acting as a clobber).
lateout(<reg>) <expr>
- Identical to
out
except that the register allocator can reuse a register allocated to anin
. - You should only write to the register after all inputs are read, otherwise you may clobber an input.
- Identical to
inout(<reg>) <expr>
<reg>
can refer to a register class or an explicit register. The allocated register name is substituted into the asm template string.- The allocated register will contain the value of
<expr>
at the start of the asm code. <expr>
must be a mutable initialized place expression, to which the contents of the allocated register is written to at the end of the asm code.
inout(<reg>) <in expr> => <out expr>
- Same as
inout
except that the initial value of the register is taken from the value of<in expr>
. <out expr>
must be a (possibly uninitialized) place expression, to which the contents of the allocated register is written to at the end of the asm code.- An underscore (
_
) may be specified instead of an expression for<out expr>
, which will cause the contents of the register to be discarded at the end of the asm code (effectively acting as a clobber). <in expr>
and<out expr>
may have different types.
- Same as
inlateout(<reg>) <expr>
/inlateout(<reg>) <in expr> => <out expr>
- Identical to
inout
except that the register allocator can reuse a register allocated to anin
(this can happen if the compiler knows thein
has the same initial value as theinlateout
). - You should only write to the register after all inputs are read, otherwise you may clobber an input.
- Identical to
const <expr>
<expr>
must be an integer constant expression.- The value of the expression is formatted as a string and substituted directly into the asm template string.
sym <path>
<path>
must refer to afn
orstatic
.- A mangled symbol name referring to the item is substituted into the asm template string.
- The substituted string does not include any modifiers (e.g. GOT, PLT, relocations, etc).
<path>
is allowed to point to a#[thread_local]
static, in which case the asm code can combine the symbol with relocations (e.g.@plt
,@TPOFF
) to read from thread-local data.
Operand expressions are evaluated from left to right, just like function call arguments. After the asm!
has executed, outputs are written to in left to right order. This is significant if two outputs point to the same place: that place will contain the value of the rightmost output.
Register operands
Input and output operands can be specified either as an explicit register or as a register class from which the register allocator can select a register. Explicit registers are specified as string literals (e.g. "eax"
) while register classes are specified as identifiers (e.g. reg
). Using string literals for register names enables support for architectures that use special characters in register names, such as MIPS ($0
, $1
, etc).
Note that explicit registers treat register aliases (e.g. r14
vs lr
on ARM) and smaller views of a register (e.g. eax
vs rax
) as equivalent to the base register. It is a compile-time error to use the same explicit register for two input operands or two output operands. Additionally, it is also a compile-time error to use overlapping registers (e.g. ARM VFP) in input operands or in output operands.
Only the following types are allowed as operands for inline assembly:
- Integers (signed and unsigned)
- Floating-point numbers
- Pointers (thin only)
- Function pointers
- SIMD vectors (structs defined with
#[repr(simd)]
and which implementCopy
). This includes architecture-specific vector types defined instd::arch
such as__m128
(x86) orint8x16_t
(ARM).
Here is the list of currently supported register classes:
Architecture | Register class | Registers | LLVM constraint code |
---|---|---|---|
x86 | reg | ax , bx , cx , dx , si , di , bp , r[8-15] (x86-64 only) | r |
x86 | reg_abcd | ax , bx , cx , dx | Q |
x86-32 | reg_byte | al , bl , cl , dl , ah , bh , ch , dh | q |
x86-64 | reg_byte * | al , bl , cl , dl , sil , dil , bpl , r[8-15]b | q |
x86 | xmm_reg | xmm[0-7] (x86) xmm[0-15] (x86-64) | x |
x86 | ymm_reg | ymm[0-7] (x86) ymm[0-15] (x86-64) | x |
x86 | zmm_reg | zmm[0-7] (x86) zmm[0-31] (x86-64) | v |
x86 | kreg | k[1-7] | Yk |
x86 | x87_reg | st([0-7]) | Only clobbers |
x86 | mmx_reg | mm[0-7] | Only clobbers |
AArch64 | reg | x[0-30] | r |
AArch64 | vreg | v[0-31] | w |
AArch64 | vreg_low16 | v[0-15] | x |
AArch64 | preg | p[0-15] , ffr | Only clobbers |
ARM | reg | r[0-12] , r14 | r |
ARM (Thumb) | reg_thumb | r[0-r7] | l |
ARM (ARM) | reg_thumb | r[0-r12] , r14 | l |
ARM | sreg | s[0-31] | t |
ARM | sreg_low16 | s[0-15] | x |
ARM | dreg | d[0-31] | w |
ARM | dreg_low16 | d[0-15] | t |
ARM | dreg_low8 | d[0-8] | x |
ARM | qreg | q[0-15] | w |
ARM | qreg_low8 | q[0-7] | t |
ARM | qreg_low4 | q[0-3] | x |
MIPS | reg | $[2-25] | r |
MIPS | freg | $f[0-31] | f |
NVPTX | reg16 | None* | h |
NVPTX | reg32 | None* | r |
NVPTX | reg64 | None* | l |
RISC-V | reg | x1 , x[5-7] , x[9-15] , x[16-31] (non-RV32E) | r |
RISC-V | freg | f[0-31] | f |
RISC-V | vreg | v[0-31] | Only clobbers |
Hexagon | reg | r[0-28] | r |
PowerPC | reg | r[0-31] | r |
PowerPC | reg_nonzero | r[1-31] | |
PowerPC | freg | f[0-31] | f |
PowerPC | cr | cr[0-7] , cr | Only clobbers |
PowerPC | xer | xer | Only clobbers |
wasm32 | local | None* | r |
BPF | reg | r[0-10] | r |
BPF | wreg | w[0-10] | w |
Note: On x86 we treat
reg_byte
differently fromreg
because the compiler can allocateal
andah
separately whereasreg
reserves the whole register.Note #2: On x86-64 the high byte registers (e.g.
ah
) are not available in thereg_byte
register class.Note #3: NVPTX doesn't have a fixed register set, so named registers are not supported.
Note #4: WebAssembly doesn't have registers, so named registers are not supported.
Note #5: Some register classes are marked as "Only clobbers" which means that they cannot be used for inputs or outputs, only clobbers of the form
out("reg") _
orlateout("reg") _
.
Additional register classes may be added in the future based on demand (e.g. MMX, x87, etc).
Each register class has constraints on which value types they can be used with. This is necessary because the way a value is loaded into a register depends on its type. For example, on big-endian systems, loading a i32x4
and a i8x16
into a SIMD register may result in different register contents even if the byte-wise memory representation of both values is identical. The availability of supported types for a particular register class may depend on what target features are currently enabled.
Architecture | Register class | Target feature | Allowed types |
---|---|---|---|
x86-32 | reg | None | i16 , i32 , f32 |
x86-64 | reg | None | i16 , i32 , f32 , i64 , f64 |
x86 | reg_byte | None | i8 |
x86 | xmm_reg | sse | i32 , f32 , i64 , f64 , i8x16 , i16x8 , i32x4 , i64x2 , f32x4 , f64x2 |
x86 | ymm_reg | avx | i32 , f32 , i64 , f64 , i8x16 , i16x8 , i32x4 , i64x2 , f32x4 , f64x2 i8x32 , i16x16 , i32x8 , i64x4 , f32x8 , f64x4 |
x86 | zmm_reg | avx512f | i32 , f32 , i64 , f64 , i8x16 , i16x8 , i32x4 , i64x2 , f32x4 , f64x2 i8x32 , i16x16 , i32x8 , i64x4 , f32x8 , f64x4 i8x64 , i16x32 , i32x16 , i64x8 , f32x16 , f64x8 |
x86 | kreg | avx512f | i8 , i16 |
x86 | kreg | avx512bw | i32 , i64 |
x86 | mmx_reg | N/A | Only clobbers |
x86 | x87_reg | N/A | Only clobbers |
AArch64 | reg | None | i8 , i16 , i32 , f32 , i64 , f64 |
AArch64 | vreg | fp | i8 , i16 , i32 , f32 , i64 , f64 , i8x8 , i16x4 , i32x2 , i64x1 , f32x2 , f64x1 , i8x16 , i16x8 , i32x4 , i64x2 , f32x4 , f64x2 |
AArch64 | preg | N/A | Only clobbers |
ARM | reg | None | i8 , i16 , i32 , f32 |
ARM | sreg | vfp2 | i32 , f32 |
ARM | dreg | vfp2 | i64 , f64 , i8x8 , i16x4 , i32x2 , i64x1 , f32x2 |
ARM | qreg | neon | i8x16 , i16x8 , i32x4 , i64x2 , f32x4 |
MIPS32 | reg | None | i8 , i16 , i32 , f32 |
MIPS32 | freg | None | f32 , f64 |
MIPS64 | reg | None | i8 , i16 , i32 , i64 , f32 , f64 |
MIPS64 | freg | None | f32 , f64 |
NVPTX | reg16 | None | i8 , i16 |
NVPTX | reg32 | None | i8 , i16 , i32 , f32 |
NVPTX | reg64 | None | i8 , i16 , i32 , f32 , i64 , f64 |
RISC-V32 | reg | None | i8 , i16 , i32 , f32 |
RISC-V64 | reg | None | i8 , i16 , i32 , f32 , i64 , f64 |
RISC-V | freg | f | f32 |
RISC-V | freg | d | f64 |
RISC-V | vreg | N/A | Only clobbers |
Hexagon | reg | None | i8 , i16 , i32 , f32 |
PowerPC | reg | None | i8 , i16 , i32 |
PowerPC | reg_nonzero | None | i8 , i16 , i32 |
PowerPC | freg | None | f32 , f64 |
PowerPC | cr | N/A | Only clobbers |
PowerPC | xer | N/A | Only clobbers |
wasm32 | local | None | i8 i16 i32 i64 f32 f64 |
BPF | reg | None | i8 i16 i32 i64 |
BPF | wreg | alu32 | i8 i16 i32 |
Note: For the purposes of the above table pointers, function pointers and
isize
/usize
are treated as the equivalent integer type (i16
/i32
/i64
depending on the target).
If a value is of a smaller size than the register it is allocated in then the upper bits of that register will have an undefined value for inputs and will be ignored for outputs. The only exception is the freg
register class on RISC-V where f32
values are NaN-boxed in a f64
as required by the RISC-V architecture.
When separate input and output expressions are specified for an inout
operand, both expressions must have the same type. The only exception is if both operands are pointers or integers, in which case they are only required to have the same size. This restriction exists because the register allocators in LLVM and GCC sometimes cannot handle tied operands with different types.
Register names
Some registers have multiple names. These are all treated by the compiler as identical to the base register name. Here is the list of all supported register aliases:
Architecture | Base register | Aliases |
---|---|---|
x86 | ax | eax , rax |
x86 | bx | ebx , rbx |
x86 | cx | ecx , rcx |
x86 | dx | edx , rdx |
x86 | si | esi , rsi |
x86 | di | edi , rdi |
x86 | bp | bpl , ebp , rbp |
x86 | sp | spl , esp , rsp |
x86 | ip | eip , rip |
x86 | st(0) | st |
x86 | r[8-15] | r[8-15]b , r[8-15]w , r[8-15]d |
x86 | xmm[0-31] | ymm[0-31] , zmm[0-31] |
AArch64 | x[0-30] | w[0-30] |
AArch64 | x29 | fp |
AArch64 | x30 | lr |
AArch64 | sp | wsp |
AArch64 | xzr | wzr |
AArch64 | v[0-31] | b[0-31] , h[0-31] , s[0-31] , d[0-31] , q[0-31] |
ARM | r[0-3] | a[1-4] |
ARM | r[4-9] | v[1-6] |
ARM | r9 | rfp |
ARM | r10 | sl |
ARM | r11 | fp |
ARM | r12 | ip |
ARM | r13 | sp |
ARM | r14 | lr |
ARM | r15 | pc |
RISC-V | x0 | zero |
RISC-V | x1 | ra |
RISC-V | x2 | sp |
RISC-V | x3 | gp |
RISC-V | x4 | tp |
RISC-V | x[5-7] | t[0-2] |
RISC-V | x8 | fp , s0 |
RISC-V | x9 | s1 |
RISC-V | x[10-17] | a[0-7] |
RISC-V | x[18-27] | s[2-11] |
RISC-V | x[28-31] | t[3-6] |
RISC-V | f[0-7] | ft[0-7] |
RISC-V | f[8-9] | fs[0-1] |
RISC-V | f[10-17] | fa[0-7] |
RISC-V | f[18-27] | fs[2-11] |
RISC-V | f[28-31] | ft[8-11] |
Hexagon | r29 | sp |
Hexagon | r30 | fr |
Hexagon | r31 | lr |
BPF | r[0-10] | w[0-10] |
Some registers cannot be used for input or output operands:
Architecture | Unsupported register | Reason |
---|---|---|
All | sp | The stack pointer must be restored to its original value at the end of an asm code block. |
All | bp (x86), x29 (AArch64), x8 (RISC-V), fr (Hexagon), $fp (MIPS) | The frame pointer cannot be used as an input or output. |
ARM | r7 or r11 | On ARM the frame pointer can be either r7 or r11 depending on the target. The frame pointer cannot be used as an input or output. |
All | si (x86-32), bx (x86-64), r6 (ARM), x19 (AArch64), r19 (Hexagon), x9 (RISC-V) | This is used internally by LLVM as a "base pointer" for functions with complex stack frames. |
x86 | k0 | This is a constant zero register which can't be modified. |
x86 | ip | This is the program counter, not a real register. |
x86 | mm[0-7] | MMX registers are not currently supported (but may be in the future). |
x86 | st([0-7]) | x87 registers are not currently supported (but may be in the future). |
AArch64 | xzr | This is a constant zero register which can't be modified. |
ARM | pc | This is the program counter, not a real register. |
ARM | r9 | This is a reserved register on some ARM targets. |
MIPS | $0 or $zero | This is a constant zero register which can't be modified. |
MIPS | $1 or $at | Reserved for assembler. |
MIPS | $26 /$k0 , $27 /$k1 | OS-reserved registers. |
MIPS | $28 /$gp | Global pointer cannot be used as inputs or outputs. |
MIPS | $ra | Return address cannot be used as inputs or outputs. |
RISC-V | x0 | This is a constant zero register which can't be modified. |
RISC-V | gp , tp | These registers are reserved and cannot be used as inputs or outputs. |
Hexagon | lr | This is the link register which cannot be used as an input or output. |
In some cases LLVM will allocate a "reserved register" for reg
operands even though this register cannot be explicitly specified. Assembly code making use of reserved registers should be careful since reg
operands may alias with those registers. Reserved registers are the frame pointer and base pointer
- The frame pointer and LLVM base pointer on all architectures.
r9
on ARM.x18
on AArch64.
Template modifiers
The placeholders can be augmented by modifiers which are specified after the :
in the curly braces. These modifiers do not affect register allocation, but change the way operands are formatted when inserted into the template string. Only one modifier is allowed per template placeholder.
The supported modifiers are a subset of LLVM's (and GCC's) asm template argument modifiers, but do not use the same letter codes.
Architecture | Register class | Modifier | Example output | LLVM modifier |
---|---|---|---|---|
x86-32 | reg | None | eax | k |
x86-64 | reg | None | rax | q |
x86-32 | reg_abcd | l | al | b |
x86-64 | reg | l | al | b |
x86 | reg_abcd | h | ah | h |
x86 | reg | x | ax | w |
x86 | reg | e | eax | k |
x86-64 | reg | r | rax | q |
x86 | reg_byte | None | al / ah | None |
x86 | xmm_reg | None | xmm0 | x |
x86 | ymm_reg | None | ymm0 | t |
x86 | zmm_reg | None | zmm0 | g |
x86 | *mm_reg | x | xmm0 | x |
x86 | *mm_reg | y | ymm0 | t |
x86 | *mm_reg | z | zmm0 | g |
x86 | kreg | None | k1 | None |
AArch64 | reg | None | x0 | x |
AArch64 | reg | w | w0 | w |
AArch64 | reg | x | x0 | x |
AArch64 | vreg | None | v0 | None |
AArch64 | vreg | v | v0 | None |
AArch64 | vreg | b | b0 | b |
AArch64 | vreg | h | h0 | h |
AArch64 | vreg | s | s0 | s |
AArch64 | vreg | d | d0 | d |
AArch64 | vreg | q | q0 | q |
ARM | reg | None | r0 | None |
ARM | sreg | None | s0 | None |
ARM | dreg | None | d0 | P |
ARM | qreg | None | q0 | q |
ARM | qreg | e / f | d0 / d1 | e / f |
MIPS | reg | None | $2 | None |
MIPS | freg | None | $f0 | None |
NVPTX | reg16 | None | rs0 | None |
NVPTX | reg32 | None | r0 | None |
NVPTX | reg64 | None | rd0 | None |
RISC-V | reg | None | x1 | None |
RISC-V | freg | None | f0 | None |
Hexagon | reg | None | r0 | None |
PowerPC | reg | None | 0 | None |
PowerPC | reg_nonzero | None | 3 | b |
PowerPC | freg | None | 0 | None |
Notes:
- on ARM
e
/f
: this prints the low or high doubleword register name of a NEON quad (128-bit) register.- on x86: our behavior for
reg
with no modifiers differs from what GCC does. GCC will infer the modifier based on the operand value type, while we default to the full register size.- on x86
xmm_reg
: thex
,t
andg
LLVM modifiers are not yet implemented in LLVM (they are supported by GCC only), but this should be a simple change.
As stated in the previous section, passing an input value smaller than the register width will result in the upper bits of the register containing undefined values. This is not a problem if the inline asm only accesses the lower bits of the register, which can be done by using a template modifier to use a subregister name in the asm code (e.g. ax
instead of rax
). Since this an easy pitfall, the compiler will suggest a template modifier to use where appropriate given the input type. If all references to an operand already have modifiers then the warning is suppressed for that operand.
ABI clobbers
The clobber_abi
keyword can be used to apply a default set of clobbers to an asm
block. This will automatically insert the necessary clobber constraints as needed for calling a function with a particular calling convention: if the calling convention does not fully preserve the value of a register across a call then a lateout("reg") _
is implicitly added to the operands list.
Generic register class outputs are disallowed by the compiler when clobber_abi
is used: all outputs must specify an explicit register. Explicit register outputs have precedence over the implicit clobbers inserted by clobber_abi
: a clobber will only be inserted for a register if that register is not used as an output.
The following ABIs can be used with clobber_abi
:
Architecture | ABI name | Clobbered registers |
---|---|---|
x86-32 | "C" , "system" , "efiapi" , "cdecl" , "stdcall" , "fastcall" | ax , cx , dx , xmm[0-7] , mm[0-7] , k[1-7] , st([0-7]) |
x86-64 | "C" , "system" (on Windows), "efiapi" , "win64" | ax , cx , dx , r[8-11] , xmm[0-31] , mm[0-7] , k[1-7] , st([0-7]) |
x86-64 | "C" , "system" (on non-Windows), "sysv64" | ax , cx , dx , si , di , r[8-11] , xmm[0-31] , mm[0-7] , k[1-7] , st([0-7]) |
AArch64 | "C" , "system" , "efiapi" | x[0-17] , x30 , v[0-31] , p[0-15] , ffr |
ARM | "C" , "system" , "efiapi" , "aapcs" | r[0-3] , r12 , r14 , s[0-15] , d[0-7] , d[16-31] |
RISC-V | "C" , "system" , "efiapi" | x1 , x[5-7] , x[10-17] , x[28-31] , f[0-7] , f[10-17] , f[28-31] , v[0-31] |
The list of clobbered registers for each ABI is updated in rustc as architectures gain new registers: this ensures that asm
clobbers will continue to be correct when LLVM starts using these new registers in its generated code.
Options
Flags are used to further influence the behavior of the inline assembly block. Currently the following options are defined:
pure
: Theasm
block has no side effects, and its outputs depend only on its direct inputs (i.e. the values themselves, not what they point to) or values read from memory (unless thenomem
options is also set). This allows the compiler to execute theasm
block fewer times than specified in the program (e.g. by hoisting it out of a loop) or even eliminate it entirely if the outputs are not used.nomem
: Theasm
blocks does not read or write to any memory. This allows the compiler to cache the values of modified global variables in registers across theasm
block since it knows that they are not read or written to by theasm
.readonly
: Theasm
block does not write to any memory. This allows the compiler to cache the values of unmodified global variables in registers across theasm
block since it knows that they are not written to by theasm
.preserves_flags
: Theasm
block does not modify the flags register (defined in the rules below). This allows the compiler to avoid recomputing the condition flags after theasm
block.noreturn
: Theasm
block never returns, and its return type is defined as!
(never). Behavior is undefined if execution falls through past the end of the asm code. Anoreturn
asm block behaves just like a function which doesn't return; notably, local variables in scope are not dropped before it is invoked.nostack
: Theasm
block does not push data to the stack, or write to the stack red-zone (if supported by the target). If this option is not used then the stack pointer is guaranteed to be suitably aligned (according to the target ABI) for a function call.att_syntax
: This option is only valid on x86, and causes the assembler to use the.att_syntax prefix
mode of the GNU assembler. Register operands are substituted in with a leading%
.raw
: This causes the template string to be parsed as a raw assembly string, with no special handling for{
and}
. This is primarily useful when including raw assembly code from an external file usinginclude_str!
.
The compiler performs some additional checks on options:
- The
nomem
andreadonly
options are mutually exclusive: it is a compile-time error to specify both. - The
pure
option must be combined with either thenomem
orreadonly
options, otherwise a compile-time error is emitted. - It is a compile-time error to specify
pure
on an asm block with no outputs or only discarded outputs (_
). - It is a compile-time error to specify
noreturn
on an asm block with outputs.
Rules for inline assembly
- Any registers not specified as inputs will contain an undefined value on entry to the asm block.
- An "undefined value" in the context of inline assembly means that the register can (non-deterministically) have any one of the possible values allowed by the architecture. Notably it is not the same as an LLVM
undef
which can have a different value every time you read it (since such a concept does not exist in assembly code).
- An "undefined value" in the context of inline assembly means that the register can (non-deterministically) have any one of the possible values allowed by the architecture. Notably it is not the same as an LLVM
- Any registers not specified as outputs must have the same value upon exiting the asm block as they had on entry, otherwise behavior is undefined.
- This only applies to registers which can be specified as an input or output. Other registers follow target-specific rules.
- Note that a
lateout
may be allocated to the same register as anin
, in which case this rule does not apply. Code should not rely on this however since it depends on the results of register allocation.
- Behavior is undefined if execution unwinds out of an asm block.
- This also applies if the assembly code calls a function which then unwinds.
- The set of memory locations that assembly code is allowed to read and write are the same as those allowed for an FFI function.
- Refer to the unsafe code guidelines for the exact rules.
- If the
readonly
option is set, then only memory reads are allowed. - If the
nomem
option is set then no reads or writes to memory are allowed. - These rules do not apply to memory which is private to the asm code, such as stack space allocated within the asm block.
- The compiler cannot assume that the instructions in the asm are the ones that will actually end up executed.
- This effectively means that the compiler must treat the
asm!
as a black box and only take the interface specification into account, not the instructions themselves. - Runtime code patching is allowed, via target-specific mechanisms (outside the scope of this RFC).
- This effectively means that the compiler must treat the
- Unless the
nostack
option is set, asm code is allowed to use stack space below the stack pointer.- On entry to the asm block the stack pointer is guaranteed to be suitably aligned (according to the target ABI) for a function call.
- You are responsible for making sure you don't overflow the stack (e.g. use stack probing to ensure you hit a guard page).
- You should adjust the stack pointer when allocating stack memory as required by the target ABI.
- The stack pointer must be restored to its original value before leaving the asm block.
- If the
noreturn
option is set then behavior is undefined if execution falls through to the end of the asm block. - If the
pure
option is set then behavior is undefined if theasm
has side-effects other than its direct outputs. Behavior is also undefined if two executions of theasm
code with the same inputs result in different outputs.- When used with the
nomem
option, "inputs" are just the direct inputs of theasm!
. - When used with the
readonly
option, "inputs" comprise the direct inputs of theasm!
and any memory that theasm!
block is allowed to read.
- When used with the
- These flags registers must be restored upon exiting the asm block if the
preserves_flags
option is set:- x86
- Status flags in
EFLAGS
(CF, PF, AF, ZF, SF, OF). - Floating-point status word (all).
- Floating-point exception flags in
MXCSR
(PE, UE, OE, ZE, DE, IE).
- Status flags in
- ARM
- Condition flags in
CPSR
(N, Z, C, V) - Saturation flag in
CPSR
(Q) - Greater than or equal flags in
CPSR
(GE). - Condition flags in
FPSCR
(N, Z, C, V) - Saturation flag in
FPSCR
(QC) - Floating-point exception flags in
FPSCR
(IDC, IXC, UFC, OFC, DZC, IOC).
- Condition flags in
- AArch64
- Condition flags (
NZCV
register). - Floating-point status (
FPSR
register).
- Condition flags (
- RISC-V
- Floating-point exception flags in
fcsr
(fflags
). - Vector extension state (
vtype
,vl
,vcsr
).
- Floating-point exception flags in
- x86
- On x86, the direction flag (DF in
EFLAGS
) is clear on entry to an asm block and must be clear on exit.- Behavior is undefined if the direction flag is set on exiting an asm block.
- The requirement of restoring the stack pointer and non-output registers to their original value only applies when exiting an
asm!
block.- This means that
asm!
blocks that never return (even if not markednoreturn
) don't need to preserve these registers. - When returning to a different
asm!
block than you entered (e.g. for context switching), these registers must contain the value they had upon entering theasm!
block that you are exiting.- You cannot exit an
asm!
block that has not been entered. Neither can you exit anasm!
block that has already been exited. - You are responsible for switching any target-specific state (e.g. thread-local storage, stack bounds).
- The set of memory locations that you may access is the intersection of those allowed by the
asm!
blocks you entered and exited.
- You cannot exit an
- This means that
- You cannot assume that an
asm!
block will appear exactly once in the output binary. The compiler is allowed to instantiate multiple copies of theasm!
block, for example when the function containing it is inlined in multiple places.
Note: As a general rule, the flags covered by
preserves_flags
are those which are not preserved when performing a function call.
assert_matches
The tracking issue for this feature is: #82775
async_stream
The tracking issue for this feature is: #79024
atomic_from_mut
The tracking issue for this feature is: #76314
atomic_mut_ptr
The tracking issue for this feature is: #66893
available_parallelism
The tracking issue for this feature is: #74479
backtrace
The tracking issue for this feature is: #53487
backtrace_frames
The tracking issue for this feature is: #79676
bench_black_box
The tracking issue for this feature is: #64102
bigint_helper_methods
The tracking issue for this feature is: #85532
binary_heap_as_slice
The tracking issue for this feature is: #83659
binary_heap_drain_sorted
The tracking issue for this feature is: #59278
binary_heap_into_iter_sorted
The tracking issue for this feature is: #59278
binary_heap_retain
The tracking issue for this feature is: #71503
bool_to_option
The tracking issue for this feature is: #80967
bound_as_ref
The tracking issue for this feature is: #80996
bound_map
The tracking issue for this feature is: #86026
box_into_boxed_slice
The tracking issue for this feature is: #71582
box_into_inner
The tracking issue for this feature is: #80437
box_into_pin
The tracking issue for this feature is: #62370
btree_drain_filter
The tracking issue for this feature is: #70530
buf_read_has_data_left
The tracking issue for this feature is: #86423
build_hasher_simple_hash_one
The tracking issue for this feature is: #86161
c_size_t
The tracking issue for this feature is: #88345
c_void_variant
This feature is internal to the Rust compiler and is not intended for general use.
can_vector
The tracking issue for this feature is: #69941
cell_filter_map
The tracking issue for this feature is: #81061
cell_leak
The tracking issue for this feature is: #69099
cell_update
The tracking issue for this feature is: #50186
cfg_accessible
The tracking issue for this feature is: #64797
cfg_eval
The tracking issue for this feature is: #82679
char_error_internals
This feature is internal to the Rust compiler and is not intended for general use.
char_indices_offset
The tracking issue for this feature is: #83871
char_internals
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
coerce_unsized
The tracking issue for this feature is: #27732
concat_idents
The tracking issue for this feature is: #29599
The concat_idents
feature adds a macro for concatenating multiple identifiers
into one identifier.
Examples
#![feature(concat_idents)] fn main() { fn foobar() -> u32 { 23 } let f = concat_idents!(foo, bar); assert_eq!(f(), 23); }
const_align_of_val
The tracking issue for this feature is: #46571
const_align_of_val_raw
The tracking issue for this feature is: #46571
const_alloc_layout
The tracking issue for this feature is: #67521
const_arguments_as_str
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
const_assert_type
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
const_assume
The tracking issue for this feature is: #76972
const_bigint_helper_methods
The tracking issue for this feature is: #85532
const_btree_new
The tracking issue for this feature is: #71835
const_caller_location
The tracking issue for this feature is: #76156
const_cell_into_inner
The tracking issue for this feature is: #78729
const_cow_is_borrowed
The tracking issue for this feature is: #65143
const_cstr_unchecked
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
const_default_impls
The tracking issue for this feature is: #87864
const_deref
The tracking issue for this feature is: #88955
const_discriminant
The tracking issue for this feature is: #69821
const_eval_select
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
const_float_bits_conv
The tracking issue for this feature is: #72447
const_float_classify
The tracking issue for this feature is: #72505
const_fmt_arguments_new
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
const_format_args
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
const_heap
The tracking issue for this feature is: #79597
const_inherent_unchecked_arith
The tracking issue for this feature is: #85122
const_int_unchecked_arith
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
const_intrinsic_copy
The tracking issue for this feature is: #80697
const_intrinsic_forget
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
const_intrinsic_raw_eq
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
const_io_structs
The tracking issue for this feature is: #78812
const_ip
The tracking issue for this feature is: #76205
const_ipv4
The tracking issue for this feature is: #76205
const_ipv6
The tracking issue for this feature is: #76205
const_likely
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
const_maybe_uninit_as_ptr
The tracking issue for this feature is: #75251
const_maybe_uninit_assume_init
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
const_maybe_uninit_write
The tracking issue for this feature is: #63567
const_nonnull_slice_from_raw_parts
The tracking issue for this feature is: #71941
const_num_from_num
The tracking issue for this feature is: #87852
const_option
The tracking issue for this feature is: #67441
const_pin
The tracking issue for this feature is: #76654
const_pref_align_of
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
const_ptr_is_null
The tracking issue for this feature is: #74939
const_ptr_offset
The tracking issue for this feature is: #71499
const_ptr_offset_from
The tracking issue for this feature is: #41079
const_ptr_read
The tracking issue for this feature is: #80377
const_ptr_write
The tracking issue for this feature is: #86302
const_raw_ptr_comparison
The tracking issue for this feature is: #53020
const_replace
The tracking issue for this feature is: #83164
const_result
The tracking issue for this feature is: #82814
const_size_of_val
The tracking issue for this feature is: #46571
const_size_of_val_raw
The tracking issue for this feature is: #46571
const_slice_first_last
The tracking issue for this feature is: #83570
const_slice_from_raw_parts
The tracking issue for this feature is: #67456
const_slice_ptr_len
The tracking issue for this feature is: #71146
const_socketaddr
The tracking issue for this feature is: #82485
const_swap
The tracking issue for this feature is: #83163
const_transmute_copy
The tracking issue for this feature is: #83165
const_type_id
The tracking issue for this feature is: #77125
const_type_name
The tracking issue for this feature is: #63084
const_unsafecell_get_mut
The tracking issue for this feature is: #88836
container_error_extra
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
control_flow_enum
The tracking issue for this feature is: #75744
convert_float_to_int
The tracking issue for this feature is: #67057
core_intrinsics
This feature is internal to the Rust compiler and is not intended for general use.
core_panic
This feature is internal to the Rust compiler and is not intended for general use.
core_private_bignum
This feature is internal to the Rust compiler and is not intended for general use.
core_private_diy_float
This feature is internal to the Rust compiler and is not intended for general use.
cow_is_borrowed
The tracking issue for this feature is: #65143
cstring_from_vec_with_nul
The tracking issue for this feature is: #73179
cursor_remaining
The tracking issue for this feature is: #86369
deadline_api
The tracking issue for this feature is: #46316
dec2flt
This feature is internal to the Rust compiler and is not intended for general use.
default_free_fn
The tracking issue for this feature is: #73014
Adds a free default()
function to the std::default
module. This function
just forwards to Default::default()
, but may remove repetition of the word
"default" from the call site.
Here is an example:
#![feature(default_free_fn)] use std::default::default; #[derive(Default)] struct AppConfig { foo: FooConfig, bar: BarConfig, } #[derive(Default)] struct FooConfig { foo: i32, } #[derive(Default)] struct BarConfig { bar: f32, baz: u8, } fn main() { let options = AppConfig { foo: default(), bar: BarConfig { bar: 10.1, ..default() }, }; }
derive_clone_copy
This feature is internal to the Rust compiler and is not intended for general use.
derive_eq
This feature is internal to the Rust compiler and is not intended for general use.
dir_entry_ext2
The tracking issue for this feature is: #85573
discriminant_kind
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
dispatch_from_dyn
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
div_duration
The tracking issue for this feature is: #63139
drain_filter
The tracking issue for this feature is: #43244
duration_checked_float
The tracking issue for this feature is: #83400
duration_constants
The tracking issue for this feature is: #57391
duration_consts_2
The tracking issue for this feature is: #72440
edition_panic
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
entry_insert
The tracking issue for this feature is: #65225
error_iter
The tracking issue for this feature is: #58520
error_type_id
The tracking issue for this feature is: #60784
exact_size_is_empty
The tracking issue for this feature is: #35428
exit_status_error
The tracking issue for this feature is: #84908
extend_one
The tracking issue for this feature is: #72631
fd
This feature is internal to the Rust compiler and is not intended for general use.
fd_read
This feature is internal to the Rust compiler and is not intended for general use.
float_interpolation
The tracking issue for this feature is: #86269
flt2dec
This feature is internal to the Rust compiler and is not intended for general use.
fmt_internals
This feature is internal to the Rust compiler and is not intended for general use.
fn_traits
The tracking issue for this feature is #29625
See Also: unboxed_closures
The fn_traits
feature allows for implementation of the Fn*
traits
for creating custom closure-like types.
#![feature(unboxed_closures)] #![feature(fn_traits)] struct Adder { a: u32 } impl FnOnce<(u32, )> for Adder { type Output = u32; extern "rust-call" fn call_once(self, b: (u32, )) -> Self::Output { self.a + b.0 } } fn main() { let adder = Adder { a: 3 }; assert_eq!(adder(2), 5); }
forget_unsized
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
format_args_nl
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
future_poll_fn
The tracking issue for this feature is: #72302
gen_future
The tracking issue for this feature is: #50547
generator_trait
The tracking issue for this feature is: #43122
get_mut_unchecked
The tracking issue for this feature is: #63292
global_asm
The tracking issue for this feature is: #35119
The global_asm!
macro allows the programmer to write arbitrary
assembly outside the scope of a function body, passing it through
rustc
and llvm
to the assembler. That is to say, global_asm!
is
equivalent to assembling the asm with an external assembler and then
linking the resulting object file with the current crate.
global_asm!
fills a role not currently satisfied by either asm!
or #[naked]
functions. The programmer has all features of the
assembler at their disposal. The linker will expect to resolve any
symbols defined in the inline assembly, modulo any symbols marked as
external. It also means syntax for directives and assembly follow the
conventions of the assembler in your toolchain.
A simple usage looks like this:
#![feature(global_asm)]
// you also need relevant target_arch cfgs
global_asm!(include_str!("something_neato.s"));
And a more complicated usage looks like this:
#![allow(unused)] #![feature(global_asm)] fn main() { #[cfg(any(target_arch="x86", target_arch="x86_64"))] mod x86 { pub mod sally { global_asm!( ".global foo", "foo:", "jmp baz", ); #[no_mangle] pub unsafe extern "C" fn baz() {} } // the symbols `foo` and `bar` are global, no matter where // `global_asm!` was used. extern "C" { fn foo(); fn bar(); } pub mod harry { global_asm!( ".global bar", "bar:", "jmp quux", ); #[no_mangle] pub unsafe extern "C" fn quux() {} } } }
You may use global_asm!
multiple times, anywhere in your crate, in
whatever way suits you. However, you should not rely on assembler state
(e.g. assembler macros) defined in one global_asm!
to be available in
another one. It is implementation-defined whether the multiple usages
are concatenated into one or assembled separately.
global_asm!
also supports const
operands like asm!
, which allows
constants defined in Rust to be used in assembly code:
#![allow(unused)] #![feature(global_asm)] fn main() { #[cfg(any(target_arch="x86", target_arch="x86_64"))] mod x86 { const C: i32 = 1234; global_asm!( ".global bar", "bar: .word {c}", c = const C, ); } }
The syntax for passing operands is the same as asm!
except that only
const
operands are allowed. Refer to the asm documentation
for more details.
On x86, the assembly code will use intel syntax by default. You can
override this by adding options(att_syntax)
at the end of the macro
arguments list:
#![allow(unused)] #![feature(global_asm)] fn main() { #[cfg(any(target_arch="x86", target_arch="x86_64"))] mod x86 { global_asm!("movl ${}, %ecx", const 5, options(att_syntax)); // is equivalent to global_asm!("mov ecx, {}", const 5); } }
If you don't need quite as much power and flexibility as
global_asm!
provides, and you don't mind restricting your inline
assembly to fn
bodies only, you might try the
asm feature instead.
hash_drain_filter
The tracking issue for this feature is: #59618
hash_raw_entry
The tracking issue for this feature is: #56167
hash_set_entry
The tracking issue for this feature is: #60896
hashmap_internals
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
inherent_ascii_escape
The tracking issue for this feature is: #77174
inplace_iteration
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
int_abs_diff
The tracking issue for this feature is: #89492
int_error_internals
This feature is internal to the Rust compiler and is not intended for general use.
int_log
The tracking issue for this feature is: #70887
int_roundings
The tracking issue for this feature is: #88581
integer_atomics
The tracking issue for this feature is: #32976
internal_output_capture
This feature is internal to the Rust compiler and is not intended for general use.
into_future
The tracking issue for this feature is: #67644
io_error_more
The tracking issue for this feature is: #86442
io_error_uncategorized
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
io_read_to_string
The tracking issue for this feature is: #80218
io_safety
The tracking issue for this feature is: #87074
io_slice_advance
The tracking issue for this feature is: #62726
ip
The tracking issue for this feature is: #27709
is_sorted
The tracking issue for this feature is: #53485
Add the methods is_sorted
, is_sorted_by
and is_sorted_by_key
to [T]
;
add the methods is_sorted
, is_sorted_by
and is_sorted_by_key
to
Iterator
.
is_symlink
The tracking issue for this feature is: #85748
iter_advance_by
The tracking issue for this feature is: #77404
iter_intersperse
The tracking issue for this feature is: #79524
iter_is_partitioned
The tracking issue for this feature is: #62544
iter_order_by
The tracking issue for this feature is: #64295
iter_partition_in_place
The tracking issue for this feature is: #62543
iter_zip
The tracking issue for this feature is: #83574
layout_for_ptr
The tracking issue for this feature is: #69835
liballoc_internals
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
libstd_sys_internals
This feature is internal to the Rust compiler and is not intended for general use.
libstd_thread_internals
This feature is internal to the Rust compiler and is not intended for general use.
linked_list_cursors
The tracking issue for this feature is: #58533
linked_list_remove
The tracking issue for this feature is: #69210
linux_pidfd
The tracking issue for this feature is: #82971
llvm_asm
The tracking issue for this feature is: #70173
For extremely low-level manipulations and performance reasons, one
might wish to control the CPU directly. Rust supports using inline
assembly to do this via the llvm_asm!
macro.
llvm_asm!(assembly template
: output operands
: input operands
: clobbers
: options
);
Any use of llvm_asm
is feature gated (requires #![feature(llvm_asm)]
on the
crate to allow) and of course requires an unsafe
block.
Note: the examples here are given in x86/x86-64 assembly, but all platforms are supported.
Assembly template
The assembly template
is the only required parameter and must be a
literal string (i.e. ""
)
#![feature(llvm_asm)] #[cfg(any(target_arch = "x86", target_arch = "x86_64"))] fn foo() { unsafe { llvm_asm!("NOP"); } } // Other platforms: #[cfg(not(any(target_arch = "x86", target_arch = "x86_64")))] fn foo() { /* ... */ } fn main() { // ... foo(); // ... }
(The feature(llvm_asm)
and #[cfg]
s are omitted from now on.)
Output operands, input operands, clobbers and options are all optional
but you must add the right number of :
if you skip them:
#![feature(llvm_asm)] #[cfg(any(target_arch = "x86", target_arch = "x86_64"))] fn main() { unsafe { llvm_asm!("xor %eax, %eax" : : : "eax" ); } } #[cfg(not(any(target_arch = "x86", target_arch = "x86_64")))] fn main() {}
Whitespace also doesn't matter:
#![feature(llvm_asm)] #[cfg(any(target_arch = "x86", target_arch = "x86_64"))] fn main() { unsafe { llvm_asm!("xor %eax, %eax" ::: "eax"); } } #[cfg(not(any(target_arch = "x86", target_arch = "x86_64")))] fn main() {}
Operands
Input and output operands follow the same format: : "constraints1"(expr1), "constraints2"(expr2), ..."
. Output operand
expressions must be mutable place, or not yet assigned:
#![feature(llvm_asm)] #[cfg(any(target_arch = "x86", target_arch = "x86_64"))] fn add(a: i32, b: i32) -> i32 { let c: i32; unsafe { llvm_asm!("add $2, $0" : "=r"(c) : "0"(a), "r"(b) ); } c } #[cfg(not(any(target_arch = "x86", target_arch = "x86_64")))] fn add(a: i32, b: i32) -> i32 { a + b } fn main() { assert_eq!(add(3, 14159), 14162) }
If you would like to use real operands in this position, however,
you are required to put curly braces {}
around the register that
you want, and you are required to put the specific size of the
operand. This is useful for very low level programming, where
which register you use is important:
#![allow(unused)] fn main() { #![feature(llvm_asm)] #[cfg(any(target_arch = "x86", target_arch = "x86_64"))] unsafe fn read_byte_in(port: u16) -> u8 { let result: u8; llvm_asm!("in %dx, %al" : "={al}"(result) : "{dx}"(port)); result } }
Clobbers
Some instructions modify registers which might otherwise have held different values so we use the clobbers list to indicate to the compiler not to assume any values loaded into those registers will stay valid.
#![feature(llvm_asm)] #[cfg(any(target_arch = "x86", target_arch = "x86_64"))] fn main() { unsafe { // Put the value 0x200 in eax: llvm_asm!("mov $$0x200, %eax" : /* no outputs */ : /* no inputs */ : "eax"); } } #[cfg(not(any(target_arch = "x86", target_arch = "x86_64")))] fn main() {}
Input and output registers need not be listed since that information is already communicated by the given constraints. Otherwise, any other registers used either implicitly or explicitly should be listed.
If the assembly changes the condition code register cc
should be
specified as one of the clobbers. Similarly, if the assembly modifies
memory, memory
should also be specified.
Options
The last section, options
is specific to Rust. The format is comma
separated literal strings (i.e. :"foo", "bar", "baz"
). It's used to
specify some extra info about the inline assembly:
Current valid options are:
volatile
- specifying this is analogous to__asm__ __volatile__ (...)
in gcc/clang.alignstack
- certain instructions expect the stack to be aligned a certain way (i.e. SSE) and specifying this indicates to the compiler to insert its usual stack alignment codeintel
- use intel syntax instead of the default AT&T.
#![feature(llvm_asm)] #[cfg(any(target_arch = "x86", target_arch = "x86_64"))] fn main() { let result: i32; unsafe { llvm_asm!("mov eax, 2" : "={eax}"(result) : : : "intel") } println!("eax is currently {}", result); } #[cfg(not(any(target_arch = "x86", target_arch = "x86_64")))] fn main() {}
More Information
The current implementation of the llvm_asm!
macro is a direct binding to LLVM's
inline assembler expressions, so be sure to check out their
documentation as well for more information about clobbers,
constraints, etc.
If you need more power and don't mind losing some of the niceties of
llvm_asm!
, check out global_asm.
log_syntax
The tracking issue for this feature is: #29598
map_entry_replace
The tracking issue for this feature is: #44286
map_first_last
The tracking issue for this feature is: #62924
map_try_insert
The tracking issue for this feature is: #82766
maybe_uninit_array_assume_init
The tracking issue for this feature is: #80908
maybe_uninit_extra
The tracking issue for this feature is: #63567
maybe_uninit_slice
The tracking issue for this feature is: #63569
maybe_uninit_uninit_array
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
maybe_uninit_write_slice
The tracking issue for this feature is: #79995
mixed_integer_ops
The tracking issue for this feature is: #87840
mutex_unlock
The tracking issue for this feature is: #81872
new_uninit
The tracking issue for this feature is: #63291
nonnull_slice_from_raw_parts
The tracking issue for this feature is: #71941
nonzero_is_power_of_two
The tracking issue for this feature is: #81106
nonzero_ops
The tracking issue for this feature is: #84186
numfmt
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
once_cell
The tracking issue for this feature is: #74465
option_get_or_insert_default
The tracking issue for this feature is: #82901
option_result_contains
The tracking issue for this feature is: #62358
option_result_unwrap_unchecked
The tracking issue for this feature is: #81383
option_zip
The tracking issue for this feature is: #70086
panic_abort
The tracking issue for this feature is: #32837
panic_always_abort
The tracking issue for this feature is: #84438
panic_info_message
The tracking issue for this feature is: #66745
panic_internals
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
panic_unwind
The tracking issue for this feature is: #32837
path_file_prefix
The tracking issue for this feature is: #86319
path_try_exists
The tracking issue for this feature is: #83186
pattern
The tracking issue for this feature is: #27721
peer_credentials_unix_socket
The tracking issue for this feature is: #42839
pin_deref_mut
The tracking issue for this feature is: #86918
pin_static_ref
The tracking issue for this feature is: #78186
poll_ready
The tracking issue for this feature is: #89780
print_internals
This feature is internal to the Rust compiler and is not intended for general use.
proc_macro_def_site
The tracking issue for this feature is: #54724
proc_macro_diagnostic
The tracking issue for this feature is: #54140
proc_macro_internals
The tracking issue for this feature is: #27812
proc_macro_quote
The tracking issue for this feature is: #54722
proc_macro_span
The tracking issue for this feature is: #54725
proc_macro_span_shrink
The tracking issue for this feature is: #87552
proc_macro_tracked_env
The tracking issue for this feature is: #74690
process_exitcode_placeholder
The tracking issue for this feature is: #48711
process_internals
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
profiler_runtime_lib
This feature is internal to the Rust compiler and is not intended for general use.
ptr_as_uninit
The tracking issue for this feature is: #75402
ptr_internals
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
ptr_metadata
The tracking issue for this feature is: #81513
raw_os_nonzero
The tracking issue for this feature is: #82363
raw_vec_internals
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
read_initializer
The tracking issue for this feature is: #42788
ready_macro
The tracking issue for this feature is: #70922
receiver_trait
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
restricted_std
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
result_cloned
The tracking issue for this feature is: #63168
result_contains_err
The tracking issue for this feature is: #62358
result_copied
The tracking issue for this feature is: #63168
result_flattening
The tracking issue for this feature is: #70142
result_into_ok_or_err
The tracking issue for this feature is: #82223
rt
This feature is internal to the Rust compiler and is not intended for general use.
saturating_div
The tracking issue for this feature is: #87920
saturating_int_impl
The tracking issue for this feature is: #87920
sealed
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
seek_stream_len
The tracking issue for this feature is: #59359
set_ptr_value
The tracking issue for this feature is: #75091
setgroups
The tracking issue for this feature is: #38527
sgx_platform
The tracking issue for this feature is: #56975
slice_as_chunks
The tracking issue for this feature is: #74985
slice_concat_ext
The tracking issue for this feature is: #27747
slice_concat_trait
The tracking issue for this feature is: #27747
slice_group_by
The tracking issue for this feature is: #80552
slice_index_methods
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
slice_internals
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
slice_partition_at_index
The tracking issue for this feature is: #55300
slice_partition_dedup
The tracking issue for this feature is: #54279
slice_pattern
The tracking issue for this feature is: #56345
slice_ptr_get
The tracking issue for this feature is: #74265
slice_ptr_len
The tracking issue for this feature is: #71146
slice_range
The tracking issue for this feature is: #76393
slice_split_at_unchecked
The tracking issue for this feature is: #76014
slice_swap_unchecked
The tracking issue for this feature is: #88539
solid_ext
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
sort_internals
This feature is internal to the Rust compiler and is not intended for general use.
std_internals
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
stdin_forwarders
The tracking issue for this feature is: #87096
stdio_locked
The tracking issue for this feature is: #86845
stdsimd
The tracking issue for this feature is: #48556
step_trait
The tracking issue for this feature is: #42168
str_internals
This feature is internal to the Rust compiler and is not intended for general use.
str_split_as_str
The tracking issue for this feature is: #77998
str_split_inclusive_as_str
The tracking issue for this feature is: #77998
str_split_whitespace_as_str
The tracking issue for this feature is: #77998
stream_from_iter
The tracking issue for this feature is: #81798
string_extend_from_within
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
string_remove_matches
The tracking issue for this feature is: #72826
tcp_linger
The tracking issue for this feature is: #88494
tcplistener_into_incoming
The tracking issue for this feature is: #88339
termination_trait_lib
The tracking issue for this feature is: #43301
test
The tracking issue for this feature is: None.
The internals of the test
crate are unstable, behind the test
flag. The
most widely used part of the test
crate are benchmark tests, which can test
the performance of your code. Let's make our src/lib.rs
look like this
(comments elided):
#![allow(unused)] #![feature(test)] fn main() { extern crate test; pub fn add_two(a: i32) -> i32 { a + 2 } #[cfg(test)] mod tests { use super::*; use test::Bencher; #[test] fn it_works() { assert_eq!(4, add_two(2)); } #[bench] fn bench_add_two(b: &mut Bencher) { b.iter(|| add_two(2)); } } }
Note the test
feature gate, which enables this unstable feature.
We've imported the test
crate, which contains our benchmarking support.
We have a new function as well, with the bench
attribute. Unlike regular
tests, which take no arguments, benchmark tests take a &mut Bencher
. This
Bencher
provides an iter
method, which takes a closure. This closure
contains the code we'd like to benchmark.
We can run benchmark tests with cargo bench
:
$ cargo bench
Compiling adder v0.0.1 (file:///home/steve/tmp/adder)
Running target/release/adder-91b3e234d4ed382a
running 2 tests
test tests::it_works ... ignored
test tests::bench_add_two ... bench: 1 ns/iter (+/- 0)
test result: ok. 0 passed; 0 failed; 1 ignored; 1 measured
Our non-benchmark test was ignored. You may have noticed that cargo bench
takes a bit longer than cargo test
. This is because Rust runs our benchmark
a number of times, and then takes the average. Because we're doing so little
work in this example, we have a 1 ns/iter (+/- 0)
, but this would show
the variance if there was one.
Advice on writing benchmarks:
- Move setup code outside the
iter
loop; only put the part you want to measure inside - Make the code do "the same thing" on each iteration; do not accumulate or change state
- Make the outer function idempotent too; the benchmark runner is likely to run it many times
- Make the inner
iter
loop short and fast so benchmark runs are fast and the calibrator can adjust the run-length at fine resolution - Make the code in the
iter
loop do something simple, to assist in pinpointing performance improvements (or regressions)
Gotcha: optimizations
There's another tricky part to writing benchmarks: benchmarks compiled with optimizations activated can be dramatically changed by the optimizer so that the benchmark is no longer benchmarking what one expects. For example, the compiler might recognize that some calculation has no external effects and remove it entirely.
#![allow(unused)] #![feature(test)] fn main() { extern crate test; use test::Bencher; #[bench] fn bench_xor_1000_ints(b: &mut Bencher) { b.iter(|| { (0..1000).fold(0, |old, new| old ^ new); }); } }
gives the following results
running 1 test
test bench_xor_1000_ints ... bench: 0 ns/iter (+/- 0)
test result: ok. 0 passed; 0 failed; 0 ignored; 1 measured
The benchmarking runner offers two ways to avoid this. Either, the closure that
the iter
method receives can return an arbitrary value which forces the
optimizer to consider the result used and ensures it cannot remove the
computation entirely. This could be done for the example above by adjusting the
b.iter
call to
#![allow(unused)] fn main() { struct X; impl X { fn iter<T, F>(&self, _: F) where F: FnMut() -> T {} } let b = X; b.iter(|| { // Note lack of `;` (could also use an explicit `return`). (0..1000).fold(0, |old, new| old ^ new) }); }
Or, the other option is to call the generic test::black_box
function, which
is an opaque "black box" to the optimizer and so forces it to consider any
argument as used.
#![feature(test)] extern crate test; fn main() { struct X; impl X { fn iter<T, F>(&self, _: F) where F: FnMut() -> T {} } let b = X; b.iter(|| { let n = test::black_box(1000); (0..n).fold(0, |a, b| a ^ b) }) }
Neither of these read or modify the value, and are very cheap for small values.
Larger values can be passed indirectly to reduce overhead (e.g.
black_box(&huge_struct)
).
Performing either of the above changes gives the following benchmarking results
running 1 test
test bench_xor_1000_ints ... bench: 131 ns/iter (+/- 3)
test result: ok. 0 passed; 0 failed; 0 ignored; 1 measured
However, the optimizer can still modify a testcase in an undesirable manner even when using either of the above.
thread_id_value
The tracking issue for this feature is: #67939
thread_local_const_init
The tracking issue for this feature is: #84223
thread_local_internals
This feature is internal to the Rust compiler and is not intended for general use.
thread_spawn_unchecked
The tracking issue for this feature is: #55132
toowned_clone_into
The tracking issue for this feature is: #41263
total_cmp
The tracking issue for this feature is: #72599
trace_macros
The tracking issue for this feature is #29598.
With trace_macros
you can trace the expansion of macros in your code.
Examples
#![feature(trace_macros)] fn main() { trace_macros!(true); println!("Hello, Rust!"); trace_macros!(false); }
The cargo build
output:
note: trace_macro
--> src/main.rs:5:5
|
5 | println!("Hello, Rust!");
| ^^^^^^^^^^^^^^^^^^^^^^^^^
|
= note: expanding `println! { "Hello, Rust!" }`
= note: to `print ! ( concat ! ( "Hello, Rust!" , "\n" ) )`
= note: expanding `print! { concat ! ( "Hello, Rust!" , "\n" ) }`
= note: to `$crate :: io :: _print ( format_args ! ( concat ! ( "Hello, Rust!" , "\n" ) )
)`
Finished dev [unoptimized + debuginfo] target(s) in 0.60 secs
track_path
The tracking issue for this feature is: #73921
trusted_len
The tracking issue for this feature is: #37572
trusted_random_access
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
trusted_step
The tracking issue for this feature is: #85731
try_find
The tracking issue for this feature is: #63178
try_reserve_kind
The tracking issue for this feature is: #48043
try_trait_v2
The tracking issue for this feature is: #84277
type_name_of_val
The tracking issue for this feature is: #66359
unchecked_math
The tracking issue for this feature is: #85122
unicode_internals
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
unix_chown
The tracking issue for this feature is: #88989
unix_process_wait_more
The tracking issue for this feature is: #80695
unix_socket_abstract
The tracking issue for this feature is: #85410
unix_socket_ancillary_data
The tracking issue for this feature is: #76915
unix_socket_peek
The tracking issue for this feature is: #76923
unsize
The tracking issue for this feature is: #27732
unwrap_infallible
The tracking issue for this feature is: #61695
unzip_option
The tracking issue for this feature is: #87800
update_panic_count
This feature is internal to the Rust compiler and is not intended for general use.
variant_count
The tracking issue for this feature is: #73662
vec_into_raw_parts
The tracking issue for this feature is: #65816
vec_spare_capacity
The tracking issue for this feature is: #75017
vec_split_at_spare
The tracking issue for this feature is: #81944
wasi_ext
The tracking issue for this feature is: #71213
windows_by_handle
The tracking issue for this feature is: #63010
windows_c
This feature is internal to the Rust compiler and is not intended for general use.
windows_file_type_ext
This feature has no tracking issue, and is therefore likely internal to the compiler, not being intended for general use.
windows_handle
This feature is internal to the Rust compiler and is not intended for general use.
windows_net
This feature is internal to the Rust compiler and is not intended for general use.
windows_process_extensions_force_quotes
The tracking issue for this feature is: #82227
windows_process_extensions_raw_arg
The tracking issue for this feature is: #29494
windows_stdio
This feature is internal to the Rust compiler and is not intended for general use.
with_options
The tracking issue for this feature is: #65439
wrapping_int_impl
The tracking issue for this feature is: #32463
wrapping_next_power_of_two
The tracking issue for this feature is: #32463
write_all_vectored
The tracking issue for this feature is: #70436